The article shows how to clip a cone by a plane.
The vtkClipPolyData object provides a major algorithm to process the 3D model.
Here is python script that describes all details about it.
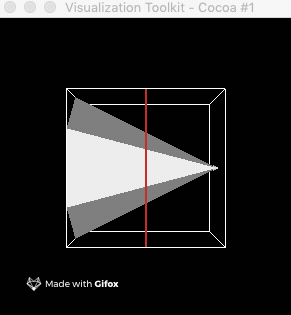
#!/usr/bin/env python
import vtk
cone = vtk.vtkConeSource()
coneMapper = vtk.vtkPolyDataMapper()
coneMapper.SetInputConnection( cone.GetOutputPort() )
coneActor = vtk.vtkActor()
coneActor.SetMapper( coneMapper )
ren1= vtk.vtkRenderer()
ren1.AddActor( coneActor )
ren1.SetBackground( 0, 0, 0 )
renWin = vtk.vtkRenderWindow()
renWin.AddRenderer( ren1 ) # selection part - green color part
plane = vtk.vtkPlane()
clipper = vtk.vtkClipPolyData()
clipper.SetInputConnection( cone.GetOutputPort() )
clipper.SetClipFunction( plane )
clipper.InsideOutOn()
selectMapper = vtk.vtkPolyDataMapper()
selectMapper.SetInputConnection( clipper.GetOutputPort() )
selectActor = vtk.vtkLODActor()
selectActor.SetMapper(selectMapper)
selectActor.GetProperty().SetColor(0, 1, 0)
selectActor.SetScale(1.01, 1.01, 1.01)
ren1.AddActor( selectActor )# selection part end
renWinInteractor = vtk.vtkRenderWindowInteractor()
renWinInteractor.SetRenderWindow( renWin )
def myCallback(obj, event):
global plane, selectActor
obj.GetPlane(plane)
selectActor.VisibilityOn()
# ImplicitPlaneWidget - outlines and plane
planeWidget = vtk.vtkImplicitPlaneWidget()
planeWidget.SetInteractor( renWinInteractor )
planeWidget.SetPlaceFactor( 1 )
planeWidget.SetInputConnection(cone.GetOutputPort())
planeWidget.PlaceWidget()
planeWidget.AddObserver("InteractionEvent", myCallback)
planeWidget.On() # ImplicitPlaneWidget end
renWinInteractor.Start()