Open An URL On A New Browser Tab
We need to import the module web browser to open a browser tab to read the HTML page.
import webbrowser
if __name__ == "__main__":
webbrowser.open( 'https://www.weiy.city' )
Download Web Page As Text
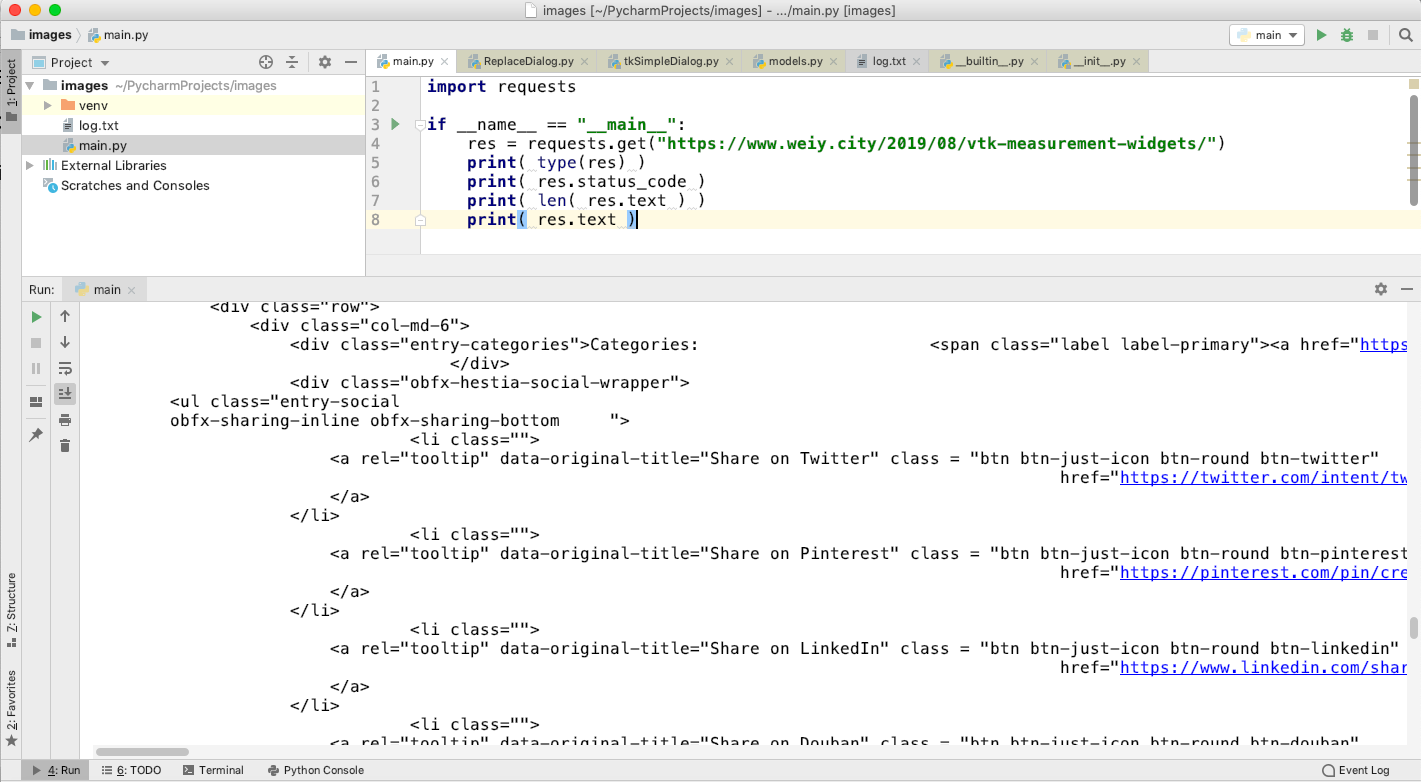
We can download the web page and write the content into a local file by multiple text chunks, it will help us to avoid too much memory occupying.
The function raise_for_status
can throw an exception if there is any problem in our project.
import requests
if __name__ == "__main__":
res = requests.get("https://www.weiy.city/2019/08/vtk-measurement-widgets/")
res.raise_for_status()
pageFile = open('page.txt', 'wb')
for chunk in res.iter_content( 10000 ):
pageFile.write( chunk )
pageFile.close()
Open Multiple URLs With Browser
I fetch HTML content from my site and open five URLs in it with multiple browser tabs. It is absolutely faster than operating manually.
import requests, sys, webbrowser, bs4
if __name__ == "__main__":
res = requests.get( "https://www.weiy.city" )
res.raise_for_status()
soup = bs4.BeautifulSoup( res.text, features='html.parser' )
elements = soup.select( 'a' )
elements = list(set(elements))
count = min( 5, len( elements ) )
for i in range(count):
urlStr = elements[i].get('href')
if urlStr[:6] == "https:" :
print( "open: " + urlStr )
webbrowser.open( urlStr )