Read a single txt file and indent every paragraph.
myReader.py
def getText( filePath ):
with open( filePath ) as file:
content = file.read()
paragraphs = content.split( '\n' )
file.close()
newContent = ""
for para in paragraphs:
newContent = newContent + " " + para + "\n"
return newContent
main.py
import myReader
filePath = "/Users/weiyang/Desktop/test.txt"
print myReader.getText( filePath )
We get new content by the script.
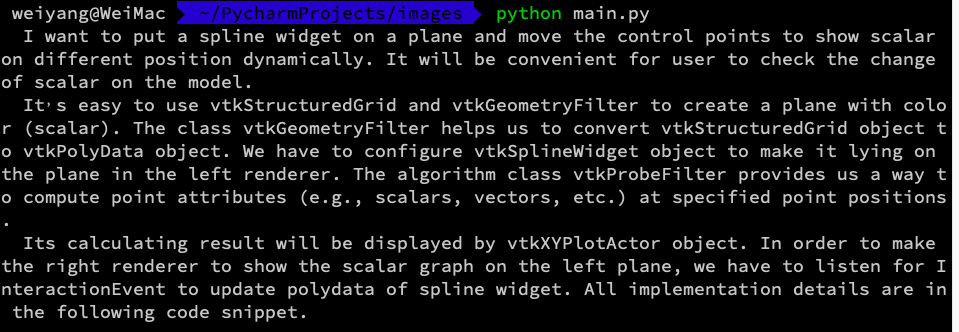
Count The Number Of Words
The script myReader.py is also used here.
import myReader
filePath = "/Users/weiyang/Desktop/test.txt"
content = myReader.getText( filePath )
content = content.rstrip()
words = content.split()
counts = dict()
for word in words:
if word in counts:
counts[word] += 1
else:
counts[word] = 1
wordMap = list()
for key,value in counts.items():
wordMap.append( (value, key) )
wordMap.sort( reverse=True ) #decrese order
for value,key in wordMap[:3]:
print ('%s \t %d' % (key, value))
