Here are some basic computing tasks for mesh in three.js.
Calculate the bounding box of mesh, set interaction style, and get the picked point.
Calculate The Bounding Box Of Mesh
mesh = new THREE.Mesh(geometry, lambertMaterial);
//...
mesh.geometry.computeBoundingBox();
const box = new THREE.Box3();
box.copy( mesh.geometry.boundingBox ).applyMatrix4( mesh.matrixWorld );
var bounds = [ box.min.x, box.max.x, box.min.y, box.max.y, box.min.z, box.max.z ];
Interaction Style
The common controller I used in my projects.
var controls;
function initControls() {
controls = new OrbitControls( camera, renderer.domElement );
// remove it if we use animate.
//controls.addEventListener( 'change', render );
// Inertia of motion
controls.enableDamping = true;
// The sensitivity of movement
controls.dampingFactor = 0.9;
controls.enableZoom = true;
controls.autoRotate = false;
controls.autoRotateSpeed = 0.5;
controls.minDistance = 0.1;
controls.maxDistance = 500;
controls.enablePan = true;
}
Animate
function render() {
renderer.render( scene, camera );
}
function animate() {
render();
controls.update();
// render using requestAnimationFrame
requestAnimationFrame(animate);
}
Get Picked Point On The 3D Model (Mesh)
function OnClicked3DPoint(evt) {
// output = document.getElementById( "webgl-output" );
var mousePosition = new THREE.Vector2();
if( evt.clientX < output.offsetLeft || evt.clientY < output.offsetTop )
{
return null;
}
evt.preventDefault();
mousePosition.x = ((evt.clientX - output.offsetLeft) / output.offsetWidth) * 2 - 1;
mousePosition.y = -((evt.clientY - output.offsetTop) / output.offsetHeight) * 2 + 1;
rayCaster.setFromCamera(mousePosition, camera);
var myObjs = new Array();
myObjs[0] = mesh;
var intersects = rayCaster.intersectObjects(myObjs, true);
/*An intersection has the following properties :
- object : intersected object (THREE.Mesh)
- distance : distance from camera to intersection (number)
- face : intersected face (THREE.Face3)
- faceIndex : intersected face index (number)
- point : intersection point (THREE.Vector3)
- uv : intersection point in the object's UV coordinates (THREE.Vector2)*/
var resultPos = null;
if (intersects.length > 0){
resultPos = intersects[0].point;
var data=[];
data = resultPos.toArray( data );
console.log( "resultPos: " + resultPos );
console.log( "data: " + data );
}
}
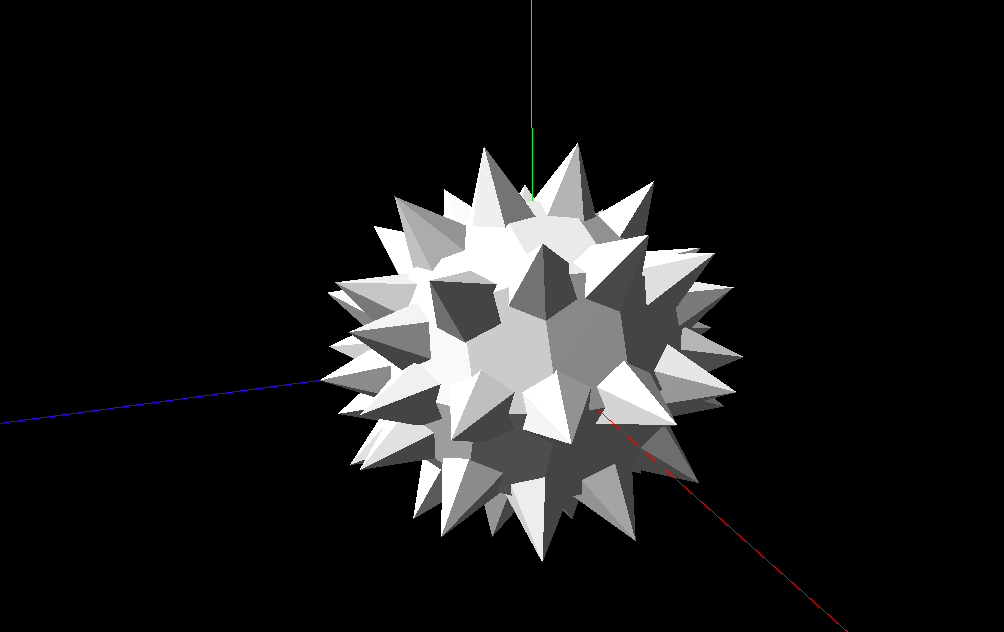