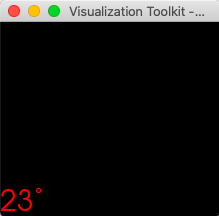
We can use vtkTextActor to show string which contains special character.
I use encoding value to represent math symbol and store it in QString object in the following example.
#include <vtkActor.h>
#include <vtkPolyData.h>
#include <vtkRenderer.h>
#include <vtkRenderWindow.h>
#include <vtkRenderWindowInteractor.h>
#include <vtkSmartPointer.h>
#include <vtkTextActor.h>
#include <vtkSphereSource.h>
#include <vtkPlaneSource.h>
#include <vtkPolyDataMapper.h>
#include <vtkPlane.h>
#include <vtkTextProperty.h>
#include <iostream>
#include <QtDebug>
#include <vector>
#include <QChar>
#include "tool.h"
int main(int, char *[])
{
QChar ch( 730 ); // Math symbol: degree
QString result = "23";
result = result + ch;
vtkSmartPointer<vtkTextActor> actor =
vtkSmartPointer<vtkTextActor>::New();
actor->SetInput( result.toStdString().c_str() );
actor->GetTextProperty()->SetFontSize( 30 );
actor->GetTextProperty()->SetColor( 1, 0, 0 );
vtkSmartPointer<vtkRenderer> renderer =
vtkSmartPointer<vtkRenderer>::New();
renderer->AddActor( actor );
renderer->SetBackground( 0, 0, 0 );
vtkSmartPointer<vtkRenderWindow> renderWindow =
vtkSmartPointer<vtkRenderWindow>::New();
renderWindow->AddRenderer( renderer );
vtkSmartPointer<vtkRenderWindowInteractor> renderWindowInteractor =
vtkSmartPointer<vtkRenderWindowInteractor>::New();
renderWindowInteractor->SetRenderWindow( renderWindow );
renderer->ResetCamera();
renderWindow->Render();
renderWindowInteractor->Start();
return EXIT_SUCCESS;
}
Lets calculate the size of renderer and the size of string, then put the string in the center of window.
int *size = renderer->GetSize();
qDebug() << size[0] << " " << size[1];
int centerY = int(size[1] / 2.0 + 0.5);
int centerX = int(size[0] / 2.0 + 0.5);
double actorSize[2];
actor->GetSize( renderer, actorSize );
actor->SetPosition( centerX - actorSize[0] / 2, centerY - actorSize[1] / 2 );
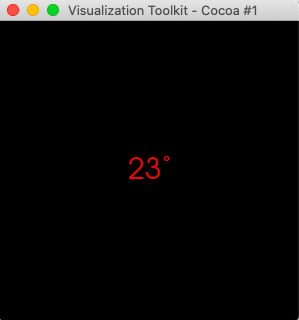
[…] put model in VTK display coordinate system. The following example is based on a previous article: Show Special Character In VTK . I add a line which start from (0,0) to left bottom corner of red string, the line is represented […]