The original image and project are in the following part.
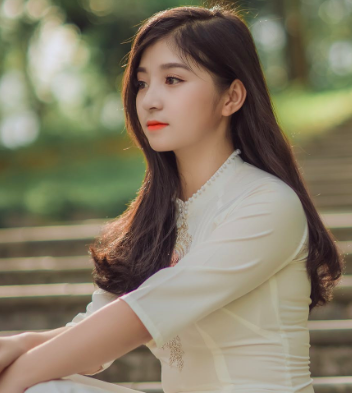
CMakeLists.txt
macro(use_cxx11)
if (CMAKE_VERSION VERSION_LESS "3.1")
if (CMAKE_CXX_COMPILER_ID STREQUAL "GNU")
set (CMAKE_CXX_FLAGS "${CMAKE_CXX_FLAGS} -std=gnu++11")
endif ()
else ()
set (CMAKE_CXX_STANDARD 11)
endif ()
endmacro(use_cxx11)
cmake_minimum_required(VERSION 2.8)
project( cvMat )
use_cxx11()
find_package( OpenCV REQUIRED )
add_executable( ${PROJECT_NAME} "main.cpp" )
target_link_libraries( ${PROJECT_NAME} ${OpenCV_LIBS} )
main.cpp
#include <stdio.h>
#include <opencv2/opencv.hpp>
using namespace cv;
int main(int argc, char** argv )
{
Mat image;
image = imread( "/Users/weiyang/Desktop/Untitled.png", 1 );
if ( !image.data )
{
printf("No image data \n");
return -1;
}
namedWindow("Display Image", WINDOW_AUTOSIZE );
imshow("Display Image", image);
waitKey(0);
return 0;
}
Convert Colorful Image To Grey Image
Mat greyImage;
cvtColor(image, greyImage, COLOR_BGR2GRAY);
imshow("Display greyImage", greyImage);
imwrite( "/Users/weiyang/Desktop/gregImage.png", greyImage );
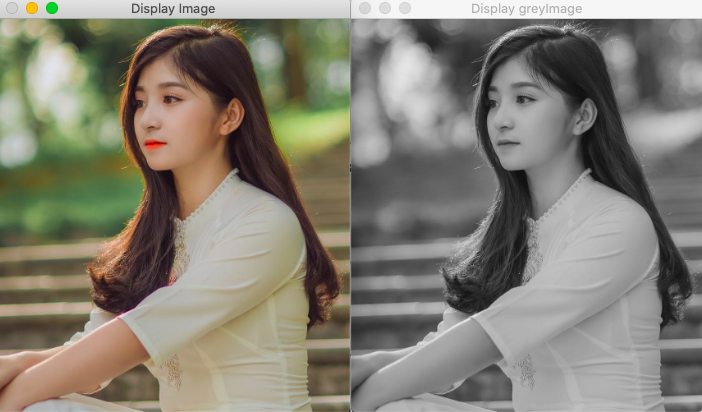
Fetch A Part Of Image
Rect region( 0, 0, image.size().width, (image.size().height + 0.5) / 2.0 );
Mat upperHalf = image( region );
imshow("Display upperHalf", upperHalf);
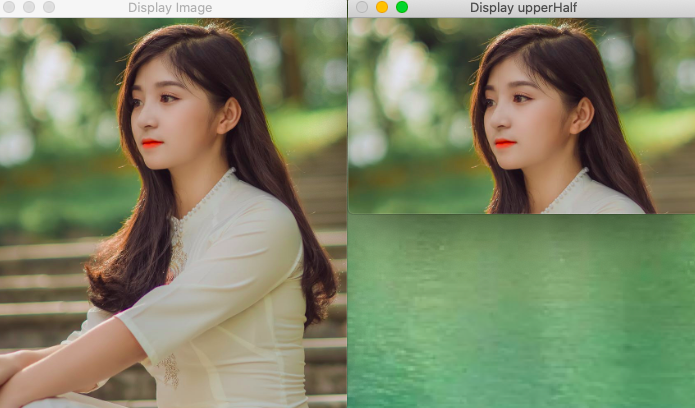
Convert To Stone Image
Mat image;
image = imread( "/Users/weiyang/Desktop/Untitled.png", 1 );
if ( !image.data )
{
printf("No image data \n");
return -1;
}
namedWindow("Display Image", WINDOW_AUTOSIZE );
imshow("Display Image", image);
Mat grey;
cvtColor(image, grey, COLOR_BGR2GRAY);
Mat sobelx;
Sobel(grey, sobelx, CV_32F, 1, 1);
namedWindow("Display sobelx", WINDOW_AUTOSIZE);
imshow("Display sobelx", sobelx);
double minVal, maxVal;
minMaxLoc(sobelx, &minVal, &maxVal); //find minimum and maximum intensities
Mat draw;
sobelx.convertTo(draw, CV_8U, 255.0/(maxVal - minVal), -minVal * 255.0/(maxVal - minVal));
namedWindow("Display draw", WINDOW_AUTOSIZE);
imshow("Display draw", draw);
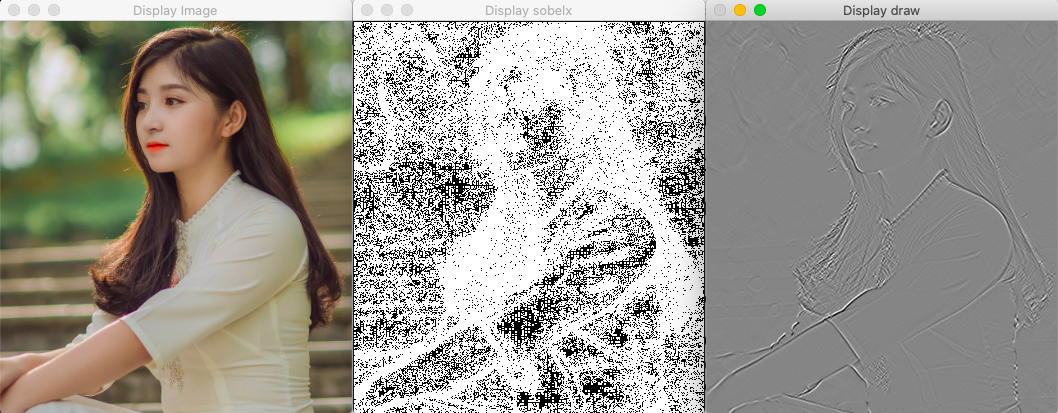