The algorithm class vtkPolyDataNormals can be used to calculate normals of points and cells in polydata.
The following example shows how to use it.
Cube
Create a cube in which every cell is a single square.
All information can be found in polydata, we use vtkPolyDataNormals to handle it and output the normals of points and cells.
Code:
#include <vtkVersion.h>
#include <vtkSmartPointer.h>
#include <vtkSurfaceReconstructionFilter.h>
#include <vtkProgrammableSource.h>
#include <vtkContourFilter.h>
#include <vtkReverseSense.h>
#include <vtkPolyDataMapper.h>
#include <vtkProperty.h>
#include <vtkPolyData.h>
#include <vtkCamera.h>
#include <vtkRenderer.h>
#include <vtkRenderWindow.h>
#include <vtkRenderWindowInteractor.h>
#include <vtkSphereSource.h>
#include <vtkXMLPolyDataReader.h>
#include <vtkUnstructuredGrid.h>
#include <vtkDataSetMapper.h>
#include <vtkPolyDataNormals.h>
#include <vtkPointData.h>
#include <vtkCellData.h>
int main(int argc, char *argv[])
{
// There are first four points which z = 0: {0, 0, 0}, {0, 0, 1}, {1, 0, 1}, {1, 0, 0}
// They form a plane
// Other points have same scene.
double coords[8][3] = { {0, 0, 0}, {0, 0, 1}, {1, 0, 1}, {1, 0, 0},
{0, 1, 0}, {0, 1, 1}, {1, 1, 1}, {1, 1, 0} };
vtkSmartPointer<vtkPoints> points =
vtkSmartPointer<vtkPoints>::New();
for( int i = 0; i < 8; ++i )
{
points->InsertPoint( i, coords[i] );
}
vtkSmartPointer<vtkCellArray> polys =
vtkSmartPointer<vtkCellArray>::New();
vtkIdType pts[6][4]={ {0,1,2,3}, {4,5,6,7}, {0,1,5,4},
{1,2,6,5}, {2,3,7,6}, {3,0,4,7} };
for( int i = 0; i < 6; ++i )
{
polys->InsertNextCell( 4, pts[i] );
}
vtkSmartPointer<vtkPolyData> pd =
vtkSmartPointer<vtkPolyData>::New();
pd->SetPolys( polys );
pd->SetPoints( points );
vtkSmartPointer<vtkPolyDataMapper> mapper =
vtkSmartPointer<vtkPolyDataMapper>::New();
mapper->SetInputData( pd );
vtkSmartPointer<vtkActor> surfaceActor =
vtkSmartPointer<vtkActor>::New();
surfaceActor->SetMapper( mapper );
vtkSmartPointer<vtkPolyDataNormals> pdNormals =
vtkSmartPointer<vtkPolyDataNormals>::New();
pdNormals->SetInputData( surfaceActor->GetMapper()->GetInput() );
pdNormals->ComputeCellNormalsOn();
pdNormals->Update();
vtkPointData* ptData = pdNormals->GetOutput()->GetPointData();
vtkDataArray* ptNormals = pdNormals->GetOutput()->GetPointData()->GetNormals();
// ostream out( std::cout.rdbuf() );
// ptData->PrintSelf( out, *vtkIndent::New() );
cout << "For points in every cell: \n";
cout << ptNormals->GetNumberOfTuples() << endl;
for( int i = 0; i < ptNormals->GetNumberOfTuples(); ++i )
{
double value[3];
ptNormals->GetTuple( i, value );
printf( "Value: (%lf, %lf, %lf)\n", value[0], value[1], value[2] );
}
cout << "For cells: \n";
printf( "pdNormals->GetOutput()->GetCellData() is %p\n",
pdNormals->GetOutput()->GetCellData() );
printf( "pdNormals->GetOutput()->GetCellData()->GetNormals() is %p\n",
pdNormals->GetOutput()->GetCellData()->GetNormals() );
if( pdNormals->GetOutput()->GetCellData() && pdNormals->GetOutput()->GetCellData()->GetNormals() )
{
vtkDataArray* cellNormals = pdNormals->GetOutput()->GetCellData()->GetNormals();
cout << cellNormals->GetNumberOfTuples() << endl;
for( int i = 0; i < cellNormals->GetNumberOfTuples(); ++i )
{
double value[3];
cellNormals->GetTuple( i, value );
printf( "Value: (%lf, %lf, %lf)\n", value[0], value[1], value[2] );
}
}
// Create the RenderWindow, Renderer and both Actors
vtkSmartPointer<vtkRenderer> ren =
vtkSmartPointer<vtkRenderer>::New();
vtkSmartPointer<vtkRenderWindow> renWin =
vtkSmartPointer<vtkRenderWindow>::New();
renWin->AddRenderer(ren);
vtkSmartPointer<vtkRenderWindowInteractor> iren =
vtkSmartPointer<vtkRenderWindowInteractor>::New();
iren->SetRenderWindow(renWin);
// Add the actors to the renderer, set the background and size
ren->AddActor( surfaceActor );
ren->SetBackground(.2, .3, .4);
renWin->Render();
iren->Start();
return EXIT_SUCCESS;
}
output:
For points in every cell:
24
Value: (0.000000, 1.000000, 0.000000)
Value: (0.000000, 1.000000, 0.000000)
Value: (0.000000, 1.000000, 0.000000)
Value: (0.000000, 1.000000, 0.000000)
Value: (0.000000, -1.000000, 0.000000)
Value: (0.000000, -1.000000, 0.000000)
Value: (0.000000, -1.000000, 0.000000)
Value: (0.000000, -1.000000, 0.000000)
Value: (1.000000, 0.000000, 0.000000)
Value: (0.000000, 0.000000, 1.000000)
Value: (1.000000, 0.000000, 0.000000)
Value: (0.000000, 0.000000, -1.000000)
Value: (0.000000, 0.000000, -1.000000)
Value: (-1.000000, 0.000000, 0.000000)
Value: (-1.000000, 0.000000, 0.000000)
Value: (0.000000, 0.000000, 1.000000)
Value: (1.000000, 0.000000, 0.000000)
Value: (0.000000, 0.000000, 1.000000)
Value: (1.000000, 0.000000, 0.000000)
Value: (0.000000, 0.000000, -1.000000)
Value: (0.000000, 0.000000, -1.000000)
Value: (-1.000000, 0.000000, 0.000000)
Value: (-1.000000, 0.000000, 0.000000)
Value: (0.000000, 0.000000, 1.000000)
For cells:
pdNormals->GetOutput()->GetCellData() is 0x7fd820f0db70
pdNormals->GetOutput()->GetCellData()->GetNormals() is 0x7fd820f10110
6
Value: (0.000000, 1.000000, 0.000000)
Value: (0.000000, -1.000000, 0.000000)
Value: (1.000000, 0.000000, 0.000000)
Value: (0.000000, 0.000000, -1.000000)
Value: (-1.000000, 0.000000, 0.000000)
Value: (0.000000, 0.000000, 1.000000)
Here are 24 output lines for points normals because the cube has 6v faces and every face has 4 vertices.
It is easy to understand why there are 6 output lines for cell normals.
Sphere
Here is a sphere example about how to use vtkPolyDataNormals.
#include <vtkVersion.h>
#include <vtkSmartPointer.h>
#include <vtkSurfaceReconstructionFilter.h>
#include <vtkProgrammableSource.h>
#include <vtkContourFilter.h>
#include <vtkReverseSense.h>
#include <vtkPolyDataMapper.h>
#include <vtkProperty.h>
#include <vtkPolyData.h>
#include <vtkCamera.h>
#include <vtkRenderer.h>
#include <vtkRenderWindow.h>
#include <vtkRenderWindowInteractor.h>
#include <vtkSphereSource.h>
#include <vtkXMLPolyDataReader.h>
#include <vtkUnstructuredGrid.h>
#include <vtkDataSetMapper.h>
#include <vtkPolyDataNormals.h>
#include <vtkPointData.h>
#include <vtkCellData.h>
int main(int argc, char *argv[])
{
vtkSmartPointer<vtkSphereSource> sphere =
vtkSmartPointer<vtkSphereSource>::New();
vtkSmartPointer<vtkPolyDataMapper> mapper =
vtkSmartPointer<vtkPolyDataMapper>::New();
mapper->SetInputConnection( sphere->GetOutputPort() );
mapper->Update();
vtkSmartPointer<vtkActor> surfaceActor =
vtkSmartPointer<vtkActor>::New();
surfaceActor->SetMapper( mapper );
vtkSmartPointer<vtkPolyDataNormals> pdNormals =
vtkSmartPointer<vtkPolyDataNormals>::New();
pdNormals->SetInputConnection( sphere->GetOutputPort() );
pdNormals->ComputeCellNormalsOn();
pdNormals->Update();
vtkPointData* ptData = pdNormals->GetOutput()->GetPointData();
printf( "ptData: %p\n", ptData );
if( ptData )
{
vtkDataArray* ptNormals = pdNormals->GetOutput()->GetPointData()->GetNormals();
printf( "ptNormals: %p\n", ptNormals );
if( ptNormals )
{
cout << "For points in every cell: \n";
cout << ptNormals->GetNumberOfTuples() << endl;
for( int i = 0; i < ptNormals->GetNumberOfTuples(); ++i )
{
double value[3];
ptNormals->GetTuple( i, value );
printf( "Value: (%lf, %lf, %lf)\n", value[0], value[1], value[2] );
}
}
}
cout << "For cells: \n";
printf( "pdNormals->GetOutput()->GetCellData() is %p\n",
pdNormals->GetOutput()->GetCellData() );
printf( "pdNormals->GetOutput()->GetCellData()->GetNormals() is %p\n",
pdNormals->GetOutput()->GetCellData()->GetNormals() );
if( pdNormals->GetOutput()->GetCellData() && pdNormals->GetOutput()->GetCellData()->GetNormals() )
{
vtkDataArray* cellNormals = pdNormals->GetOutput()->GetCellData()->GetNormals();
cout << cellNormals->GetNumberOfTuples() << endl;
for( int i = 0; i < cellNormals->GetNumberOfTuples(); ++i )
{
double value[3];
cellNormals->GetTuple( i, value );
printf( "Value: (%lf, %lf, %lf)\n", value[0], value[1], value[2] );
}
}
// Create the RenderWindow, Renderer and both Actors
vtkSmartPointer<vtkRenderer> ren =
vtkSmartPointer<vtkRenderer>::New();
vtkSmartPointer<vtkRenderWindow> renWin =
vtkSmartPointer<vtkRenderWindow>::New();
renWin->AddRenderer(ren);
vtkSmartPointer<vtkRenderWindowInteractor> iren =
vtkSmartPointer<vtkRenderWindowInteractor>::New();
iren->SetRenderWindow(renWin);
// Add the actors to the renderer, set the background and size
ren->AddActor( surfaceActor );
ren->SetBackground(.2, .3, .4);
renWin->Render();
iren->Start();
return EXIT_SUCCESS;
}
output:
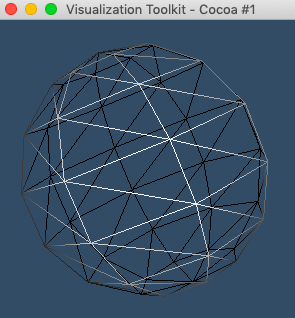
ptData: 0x7fd12b510850
ptNormals: 0x7fd12b416fc0
ptNormals: 0x7fd12b416fc0
For points in every cell:
66
Value: (0.000000, 0.000000, 1.000000)
Value: (0.000000, 0.000000, -1.000000)
Value: (0.471163, 0.052265, 0.880496)
Value: (0.779360, 0.017869, 0.626322)
Value: (0.915017, 0.379013, 0.138177)
Value: (0.887900, 0.367780, -0.276354)
Value: (0.779360, -0.017869, -0.626322)
Value: (0.471163, -0.052265, -0.880496)
Value: (0.296206, 0.370119, 0.880496)
Value: (0.538455, 0.563726, 0.626322)
Value: (0.887900, 0.367780, 0.276354)
Value: (0.915017, 0.379013, -0.138177)
Value: (0.563726, 0.538455, -0.626322)
Value: (0.370119, 0.296206, -0.880496)
Value: (-0.052265, 0.471163, 0.880496)
Value: (-0.017869, 0.779360, 0.626322)
Value: (0.367780, 0.887900, 0.276354)
Value: (0.379013, 0.915017, -0.138177)
Value: (0.017869, 0.779360, -0.626322)
Value: (0.052265, 0.471163, -0.880496)
Value: (-0.370119, 0.296206, 0.880496)
Value: (-0.563726, 0.538455, 0.626322)
Value: (-0.367780, 0.887900, 0.276354)
Value: (-0.379013, 0.915017, -0.138177)
Value: (-0.538455, 0.563726, -0.626322)
Value: (-0.296206, 0.370119, -0.880496)
Value: (-0.471163, -0.052265, 0.880496)
Value: (-0.779360, -0.017869, 0.626322)
Value: (-0.887900, 0.367780, 0.276354)
Value: (-0.915017, 0.379013, -0.138177)
Value: (-0.779360, 0.017869, -0.626322)
Value: (-0.471163, 0.052265, -0.880496)
Value: (-0.296206, -0.370119, 0.880496)
Value: (-0.538455, -0.563726, 0.626322)
Value: (-0.887900, -0.367780, 0.276354)
Value: (-0.915017, -0.379013, -0.138177)
Value: (-0.563726, -0.538455, -0.626322)
Value: (-0.370119, -0.296206, -0.880496)
Value: (0.052265, -0.471163, 0.880496)
Value: (0.017869, -0.779360, 0.626322)
Value: (-0.367780, -0.887900, 0.276354)
Value: (-0.379013, -0.915017, -0.138177)
Value: (-0.017869, -0.779360, -0.626322)
Value: (-0.052265, -0.471163, -0.880496)
Value: (0.370119, -0.296206, 0.880496)
Value: (0.563726, -0.538455, 0.626322)
Value: (0.367780, -0.887900, 0.276354)
Value: (0.379013, -0.915017, -0.138177)
Value: (0.538455, -0.563726, -0.626322)
Value: (0.296206, -0.370119, -0.880496)
Value: (0.887900, -0.367780, 0.276354)
Value: (0.915017, -0.379013, -0.138177)
Value: (0.379013, 0.915017, 0.138177)
Value: (0.367780, 0.887900, -0.276354)
Value: (-0.379013, 0.915017, 0.138177)
Value: (-0.367780, 0.887900, -0.276354)
Value: (-0.915017, 0.379013, 0.138177)
Value: (-0.887900, 0.367780, -0.276354)
Value: (-0.915017, -0.379013, 0.138177)
Value: (-0.887900, -0.367780, -0.276354)
Value: (-0.379013, -0.915017, 0.138177)
Value: (-0.367780, -0.887900, -0.276354)
Value: (0.379013, -0.915017, 0.138177)
Value: (0.367780, -0.887900, -0.276354)
Value: (0.915017, -0.379013, 0.138177)
Value: (0.887900, -0.367780, -0.276354)
For cells:
pdNormals->GetOutput()->GetCellData() is 0x7fd12b5109b0
pdNormals->GetOutput()->GetCellData()->GetNormals() is 0x7fd12b416940
96
Value: (0.221582, 0.091782, 0.970813)
Value: (0.091782, 0.221582, 0.970813)
Value: (-0.091782, 0.221582, 0.970813)
Value: (-0.221582, 0.091782, 0.970813)
Value: (-0.221582, -0.091782, 0.970813)
Value: (-0.091782, -0.221582, 0.970813)
Value: (0.091782, -0.221582, 0.970813)
Value: (0.221582, -0.091782, 0.970813)
Value: (0.221582, 0.091782, -0.970813)
Value: (0.091782, 0.221582, -0.970813)
Value: (-0.091782, 0.221582, -0.970813)
Value: (-0.221582, 0.091782, -0.970813)
Value: (-0.221582, -0.091782, -0.970813)
Value: (-0.091782, -0.221582, -0.970813)
Value: (0.091782, -0.221582, -0.970813)
Value: (0.221582, -0.091782, -0.970813)
Value: (0.603683, 0.250054, 0.756994)
Value: (0.603683, 0.250054, 0.756994)
Value: (0.844104, 0.349639, 0.406499)
Value: (0.844104, 0.349639, 0.406499)
Value: (0.923880, 0.382683, -0.000000)
Value: (0.923880, 0.382683, -0.000000)
Value: (0.844104, 0.349639, -0.406499)
Value: (0.844104, 0.349639, -0.406499)
Value: (0.603683, 0.250054, -0.756994)
Value: (0.603683, 0.250054, -0.756994)
Value: (0.250054, 0.603683, 0.756994)
Value: (0.250054, 0.603683, 0.756994)
Value: (0.349639, 0.844104, 0.406499)
Value: (0.349639, 0.844104, 0.406499)
Value: (0.382683, 0.923880, -0.000000)
Value: (0.382683, 0.923880, -0.000000)
Value: (0.349639, 0.844104, -0.406499)
Value: (0.349639, 0.844104, -0.406499)
Value: (0.250054, 0.603683, -0.756994)
Value: (0.250054, 0.603683, -0.756994)
Value: (-0.250054, 0.603683, 0.756994)
Value: (-0.250054, 0.603683, 0.756994)
Value: (-0.349639, 0.844104, 0.406499)
Value: (-0.349639, 0.844104, 0.406499)
Value: (-0.382683, 0.923880, 0.000000)
Value: (-0.382683, 0.923880, 0.000000)
Value: (-0.349639, 0.844104, -0.406499)
Value: (-0.349639, 0.844104, -0.406499)
Value: (-0.250054, 0.603683, -0.756994)
Value: (-0.250054, 0.603683, -0.756994)
Value: (-0.603683, 0.250054, 0.756994)
Value: (-0.603683, 0.250054, 0.756994)
Value: (-0.844104, 0.349639, 0.406499)
Value: (-0.844104, 0.349639, 0.406499)
Value: (-0.923880, 0.382683, 0.000000)
Value: (-0.923880, 0.382683, 0.000000)
Value: (-0.844104, 0.349639, -0.406499)
Value: (-0.844104, 0.349639, -0.406499)
Value: (-0.603683, 0.250054, -0.756994)
Value: (-0.603683, 0.250054, -0.756994)
Value: (-0.603683, -0.250054, 0.756994)
Value: (-0.603683, -0.250054, 0.756994)
Value: (-0.844104, -0.349639, 0.406499)
Value: (-0.844104, -0.349639, 0.406499)
Value: (-0.923880, -0.382683, 0.000000)
Value: (-0.923880, -0.382683, 0.000000)
Value: (-0.844104, -0.349639, -0.406499)
Value: (-0.844104, -0.349639, -0.406499)
Value: (-0.603683, -0.250054, -0.756994)
Value: (-0.603683, -0.250054, -0.756994)
Value: (-0.250054, -0.603683, 0.756994)
Value: (-0.250054, -0.603683, 0.756994)
Value: (-0.349639, -0.844104, 0.406499)
Value: (-0.349639, -0.844104, 0.406499)
Value: (-0.382683, -0.923880, 0.000000)
Value: (-0.382683, -0.923880, 0.000000)
Value: (-0.349639, -0.844104, -0.406499)
Value: (-0.349639, -0.844104, -0.406499)
Value: (-0.250054, -0.603683, -0.756994)
Value: (-0.250054, -0.603683, -0.756994)
Value: (0.250054, -0.603683, 0.756994)
Value: (0.250054, -0.603683, 0.756994)
Value: (0.349639, -0.844104, 0.406499)
Value: (0.349639, -0.844104, 0.406499)
Value: (0.382683, -0.923880, 0.000000)
Value: (0.382683, -0.923880, 0.000000)
Value: (0.349639, -0.844104, -0.406499)
Value: (0.349639, -0.844104, -0.406499)
Value: (0.250054, -0.603683, -0.756994)
Value: (0.250054, -0.603683, -0.756994)
Value: (0.603683, -0.250054, 0.756994)
Value: (0.603683, -0.250054, 0.756994)
Value: (0.844104, -0.349639, 0.406499)
Value: (0.844104, -0.349639, 0.406499)
Value: (0.923880, -0.382683, 0.000000)
Value: (0.923880, -0.382683, 0.000000)
Value: (0.844104, -0.349639, -0.406499)
Value: (0.844104, -0.349639, -0.406499)
Value: (0.603683, -0.250054, -0.756994)
Value: (0.603683, -0.250054, -0.756994)
Calculate the normals of points and show them by cones.
We use vtkGlyph3D to put cones on normals' positions.
More implementation details are showed in the following code snippet.
#include <vtkVersion.h>
#include <vtkSmartPointer.h>
#include <vtkSurfaceReconstructionFilter.h>
#include <vtkProgrammableSource.h>
#include <vtkContourFilter.h>
#include <vtkReverseSense.h>
#include <vtkPolyDataMapper.h>
#include <vtkProperty.h>
#include <vtkPolyData.h>
#include <vtkCamera.h>
#include <vtkRenderer.h>
#include <vtkRenderWindow.h>
#include <vtkRenderWindowInteractor.h>
#include <vtkSphereSource.h>
#include <vtkXMLPolyDataReader.h>
#include <vtkUnstructuredGrid.h>
#include <vtkDataSetMapper.h>
#include <vtkPolyDataNormals.h>
#include <vtkPointData.h>
#include <vtkCellData.h>
#include <vtkConeSource.h>
#include <vtkTransform.h>
#include <vtkTransformPolyDataFilter.h>
#include <vtkGlyph3D.h>
int main(int argc, char *argv[])
{
vtkSmartPointer<vtkSphereSource> sphere =
vtkSmartPointer<vtkSphereSource>::New();
vtkSmartPointer<vtkPolyDataMapper> mapper =
vtkSmartPointer<vtkPolyDataMapper>::New();
mapper->SetInputConnection( sphere->GetOutputPort() );
mapper->Update();
vtkSmartPointer<vtkActor> surfaceActor =
vtkSmartPointer<vtkActor>::New();
surfaceActor->SetMapper( mapper );
vtkSmartPointer<vtkPolyDataNormals> pdNormals =
vtkSmartPointer<vtkPolyDataNormals>::New();
pdNormals->SetInputConnection( sphere->GetOutputPort() );
pdNormals->ComputeCellNormalsOff();
pdNormals->Update();
vtkPointData* ptData = pdNormals->GetOutput()->GetPointData();
printf( "ptData: %p\n", ptData );
if( ptData )
{
vtkDataArray* ptNormals = pdNormals->GetOutput()->GetPointData()->GetNormals();
printf( "ptNormals: %p\n", ptNormals );
if( ptNormals )
{
cout << "For points in every cell: \n";
cout << ptNormals->GetNumberOfTuples() << endl;
for( int i = 0; i < ptNormals->GetNumberOfTuples(); ++i )
{
double value[3];
ptNormals->GetTuple( i, value );
printf( "Value: (%lf, %lf, %lf)\n", value[0], value[1], value[2] );
}
}
}
vtkSmartPointer<vtkConeSource> cone =
vtkSmartPointer<vtkConeSource>::New();
cone->SetResolution( 6 );
vtkSmartPointer<vtkTransform> transform =
vtkSmartPointer<vtkTransform>::New();
transform->RotateY( 180 ); // make vertex outside
vtkSmartPointer<vtkTransformPolyDataFilter> transformF =
vtkSmartPointer<vtkTransformPolyDataFilter>::New();
transformF->SetInputConnection( cone->GetOutputPort() );
transformF->SetTransform( transform );
vtkSmartPointer<vtkGlyph3D> glyph =
vtkSmartPointer<vtkGlyph3D>::New();
glyph->SetInputConnection( pdNormals->GetOutputPort() );
glyph->SetSourceConnection( transformF->GetOutputPort() ); // source => transform => graph3D
glyph->SetVectorModeToUseNormal();
glyph->SetScaleModeToScaleByVector();
glyph->SetScaleFactor( 0.1 );
vtkSmartPointer<vtkPolyDataMapper> spikeMapper =
vtkSmartPointer<vtkPolyDataMapper>::New();
spikeMapper->SetInputConnection( glyph->GetOutputPort() );
vtkSmartPointer<vtkActor> spikeActor = vtkSmartPointer<vtkActor>::New();
spikeActor->SetMapper( spikeMapper );
spikeActor->GetProperty()->SetColor( 0.0, 0.79, 0.34 );
// Create the RenderWindow, Renderer and both Actors
vtkSmartPointer<vtkRenderer> ren =
vtkSmartPointer<vtkRenderer>::New();
vtkSmartPointer<vtkRenderWindow> renWin =
vtkSmartPointer<vtkRenderWindow>::New();
renWin->AddRenderer(ren);
vtkSmartPointer<vtkRenderWindowInteractor> iren =
vtkSmartPointer<vtkRenderWindowInteractor>::New();
iren->SetRenderWindow(renWin);
// Add the actors to the renderer, set the background and size
ren->AddActor( surfaceActor );
ren->AddActor( spikeActor );
ren->SetBackground(.2, .3, .4);
renWin->Render();
iren->Start();
return EXIT_SUCCESS;
}
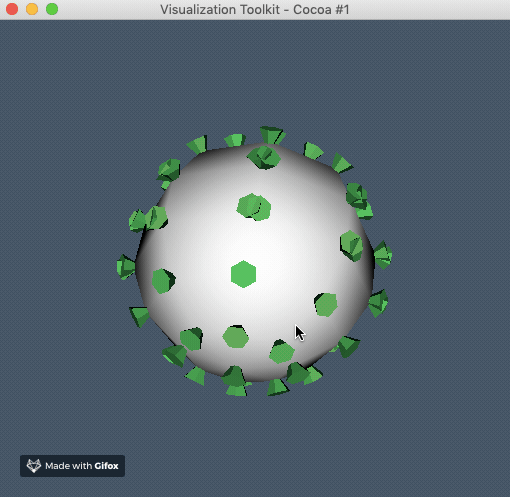
[…] post is related to The Example About vtkPolyDataNormals. If you want to use vtkPolyDataNormals to compute the normals of points, it’s better to set […]