Gamma correction is a very useful method to make the original image brighter.
It is a non-linear transform for every input value, the relevant math equation is in the following section.
O:output value
I:input value
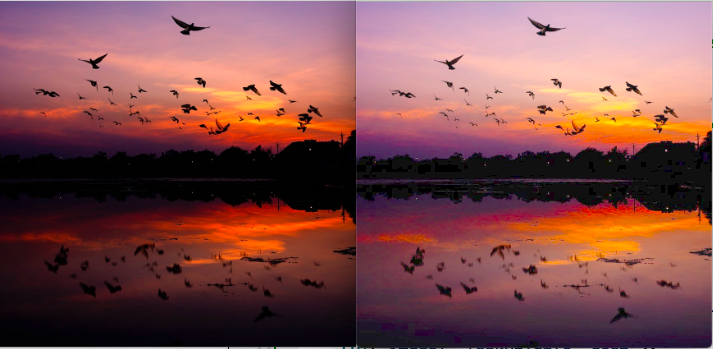
#include <stdio.h>
#include <opencv2/opencv.hpp>
using namespace cv;
int main(int argc, char** argv )
{
Mat image1 = imread( "/Users/weiyang/Desktop/p1.png", IMREAD_COLOR );
Mat dest;
if( image1.empty() )
{
fprintf( stderr, "No image data \n");
return -1;
}
double gramma_ = 0.5;
Mat lookUpTable( 1, 256, CV_8U );
uchar *p = lookUpTable.ptr();
for( int i = 0; i < 256; ++i )
{
p[i] = saturate_cast<uchar>( pow( i / 255.0, gramma_) * 255 );
}
LUT( image1, lookUpTable, dest );
namedWindow( "Display Image", WINDOW_AUTOSIZE );
imshow( "Display Image", dest );
waitKey(0);
return 0;
}
We can also use linear transform to change the original image to make it brighter.
double alpha = 1.5;
double beta = -0.3;
Mat dest = Mat::zeros( image1.size(), image1.type() );
// same as "image.convertTo(new_image, -1, alpha, beta);"
for( int r = 0; r < image1.rows; ++r )
{
for( int c = 0; c < image1.cols; ++c )
{
for( int h = 0; h < image1.channels(); ++h )
{
dest.at<Vec3b>(r, c)[h] = saturate_cast<uchar>( alpha * image1.at<Vec3b>(r, c)[h] + beta );
}
}
}
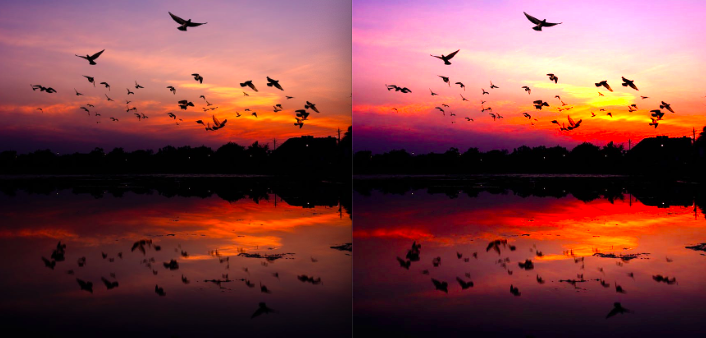