Use Transform
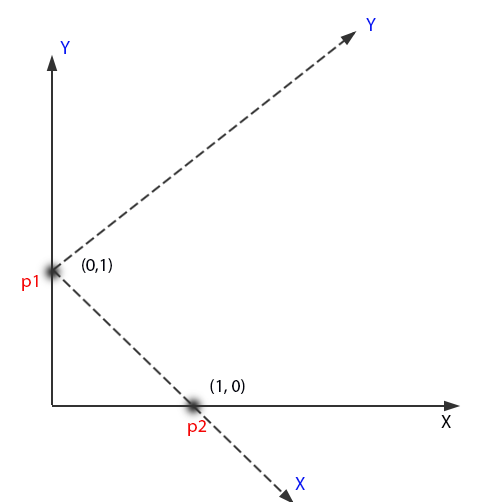
We have both points on the image, the distance weight on the x-axis between the two points is 1. What is the distance weight on the vector (p1−p2) between the two points?
It’s easy to calculate the result because we know Pythagorean theorem and the special direction is exactly p2−p1. But I write an another way to get the value here.
We can rotate the X axis to go through p1 and p2. Then the result of Xp2−Xp1 is the answer.
Code snippet:
using namespace std;
int main()
{
PointStruct p1( 0, 1, 0 );
PointStruct p2( 1, 0, 0 );
vtkSmartPointer<vtkTransform> transform =
vtkSmartPointer<vtkTransform>::New();
PointStruct v1( 1, 0, 0 );
PointStruct v2 = p2 - p1;
PointStruct rotateAxis = v1^v2;
//double angle = acos( v1.Dot( v2 ) ) * 180.0 / vtkMath::Pi();
double angle = vtkMath::AngleBetweenVectors( v1.point, v2.point );
angle = vtkMath::DegreesFromRadians( angle );
printf( "angle: %lf\n", angle );
transform->RotateWXYZ( -angle, rotateAxis.point );
transform->Update();
transform->InternalTransformPoint( p1.point, p1.point );
transform->InternalTransformPoint( p2.point, p2.point );
printf( "p1: (%lf, %lf, %lf)\n", p1[0], p1[1], p1[2] );
printf( "p2: (%lf, %lf, %lf)\n", p2[0], p2[1], p2[2] );
double result = p2[0] - p1[0];
PointStruct tmp = p2 - p1;
printf( "result: %lf\n", result );
return 0;
}
Output:
angle: 45.000000
p1: (-0.707107, 0.707107, 0.000000)
p2: (0.707107, 0.707107, 0.000000)
result: 1.414214
Create Plane
We can also create a plane which take p1 as origin and take the special vector as its normal.
Then the distance weight on the normal vector between p1 and p2 is the distance between p2 and plane.
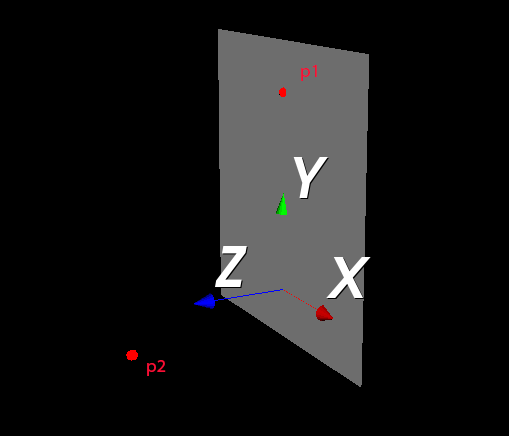
Interfaces:
vtkPlane::ProjectPoint
vtkMath::Distance2BetweenPoints
%23%23%23%20Use%20Transform%0A%0A!%5B%5D(https%3A%2F%2Fwww.weiy.city%2Fwp-content%2Fuploads%2F2020%2F01%2FUntitled1.png)%0A%0AWe%20have%20both%20points%20on%20the%20image%2C%20the%20distance%20weight%20on%20the%20x-axis%20between%20the%20two%20points%20is%201.%20What%20is%20the%20distance%20weight%20on%20the%20vector%20%24(p1%20-%20p2)%24%20between%20the%20two%20points%3F%20%20%0AIt’s%20easy%20to%20calculate%20the%20result%20because%20we%20know%20Pythagorean%20theorem%20and%20the%20special%20direction%20is%20exactly%20%24p2%20-%20p1%24.%20But%20I%20write%20an%20another%20way%20to%20get%20the%20value%20here.%0AWe%20can%20rotate%20the%20X%20axis%20to%20go%20through%20p1%20and%20p2.%20Then%20the%20result%20of%20%24X_%7Bp2%7D%20-%20X_%7Bp1%7D%24%20is%20the%20answer.%0A%0ACode%20snippet%3A%0A%0A%60%60%60cpp%0Ausing%20namespace%20std%3B%0A%0Aint%20main()%0A%7B%0A%20%20%20%20PointStruct%20p1(%200%2C%201%2C%200%20)%3B%0A%20%20%20%20PointStruct%20p2(%201%2C%200%2C%200%20)%3B%0A%20%20%20%20vtkSmartPointer%3CvtkTransform%3E%20transform%20%3D%0A%20%20%20%20%20%20%20%20%20%20%20%20vtkSmartPointer%3CvtkTransform%3E%3A%3ANew()%3B%0A%0A%20%20%20%20PointStruct%20v1(%201%2C%200%2C%200%20)%3B%0A%20%20%20%20PointStruct%20v2%20%3D%20p2%20-%20p1%3B%0A%20%20%20%20PointStruct%20rotateAxis%20%3D%20v1%5Ev2%3B%0A%20%20%20%20%2F%2Fdouble%20angle%20%3D%20acos(%20v1.Dot(%20v2%20)%20)%20*%20180.0%20%2F%20vtkMath%3A%3APi()%3B%0A%20%20%20%20double%20angle%20%3D%20vtkMath%3A%3AAngleBetweenVectors(%20v1.point%2C%20v2.point%20)%3B%0A%20%20%20%20angle%20%3D%20vtkMath%3A%3ADegreesFromRadians(%20angle%20)%3B%0A%20%20%20%20printf(%20%22angle%3A%20%25lf%5Cn%22%2C%20angle%20)%3B%0A%20%20%20%20transform-%3ERotateWXYZ(%20-angle%2C%20rotateAxis.point%20)%3B%0A%20%20%20%20transform-%3EUpdate()%3B%0A%20%20%20%20transform-%3EInternalTransformPoint(%20p1.point%2C%20p1.point%20)%3B%0A%20%20%20%20transform-%3EInternalTransformPoint(%20p2.point%2C%20p2.point%20)%3B%0A%20%20%20%20printf(%20%22p1%3A%20(%25lf%2C%20%25lf%2C%20%25lf)%5Cn%22%2C%20p1%5B0%5D%2C%20p1%5B1%5D%2C%20p1%5B2%5D%20)%3B%0A%20%20%20%20printf(%20%22p2%3A%20(%25lf%2C%20%25lf%2C%20%25lf)%5Cn%22%2C%20p2%5B0%5D%2C%20p2%5B1%5D%2C%20p2%5B2%5D%20)%3B%0A%20%20%20%20double%20result%20%3D%20p2%5B0%5D%20-%20p1%5B0%5D%3B%0A%20%20%20%20PointStruct%20tmp%20%3D%20p2%20-%20p1%3B%0A%20%20%20%20printf(%20%22result%3A%20%25lf%5Cn%22%2C%20result%20)%3B%0A%20%20%20%20return%200%3B%0A%7D%0A%60%60%60%0A%0AOutput%3A%0A%0A%60%60%60bash%0Aangle%3A%2045.000000%0Ap1%3A%20(-0.707107%2C%200.707107%2C%200.000000)%0Ap2%3A%20(0.707107%2C%200.707107%2C%200.000000)%0Aresult%3A%201.414214%0A%60%60%60%0A%0A%23%23%23%20Create%20Plane%0AWe%20can%20also%20create%20a%20plane%20which%20take%20p1%20as%20origin%20and%20take%20the%20special%20vector%20as%20its%20normal.%0AThen%20the%20distance%20weight%20on%20the%20normal%20vector%20between%20p1%20and%20p2%20is%20the%20distance%20between%20p2%20and%20plane.%0A%0A%0A!%5B%5D(https%3A%2F%2Fwww.weiy.city%2Fwp-content%2Fuploads%2F2020%2F01%2Fclip2.png)%0A%0AInterfaces%3A%0A%0A%60%60%60cpp%0AvtkPlane%3A%3AProjectPoint%0AvtkMath%3A%3ADistance2BetweenPoints%0A%60%60%60%0A%0A