The article shows how to move a model on the surface of the other big one.
I planed to move the cube on the surface of a big superquadric in the mouse left press moving event.
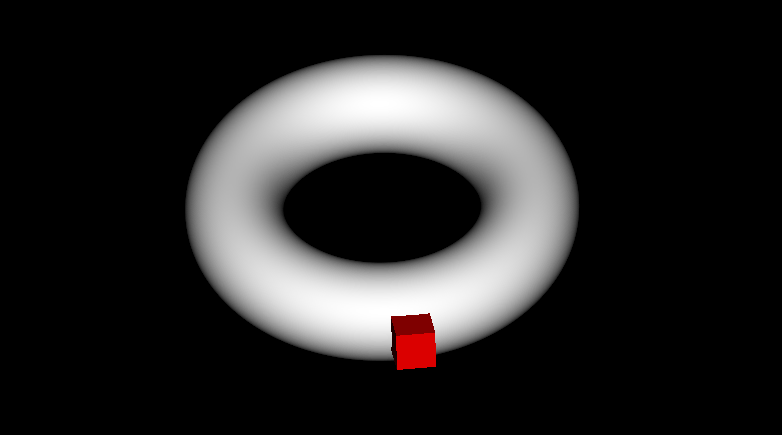
Let’s build an original scene. Created a superquadric source object in ParaView and saved it as a VTP file which can be handled by our project. The red cube was created with vtkCubeSource, then I change its position by configuring a suitable user transform.
We need a custom interactor style for the new moving operation. So I defined a class vtkCustomStyle that inherits vtkInteractorStyleTrackballCamera
.
I translate the red cube on the surface of the superquadric with the key z
matched mouse moving action.
Now let’s discuss how to make the red cube moving on the surface.
We can calculate the translating vector after vtkRenderWindowInteractor object tells us the event position and last event position. Then we can move the cube normally by multiplying the original matrix with the translating matrix. The touching matrix needs to be computed and applied if we want to fulfill the task.
I assumed the four back vertices of the red cube is on the superquadric if the cube was on the surface. The projected points which are on the surface can be regards as the target points. The source points are the original back vertices of the red cube. We can use vtkLandmarkTransform
to calculate the touching matrix and apply the result to the user transform of the red cube.
The core function for touching move is vtkCustomStyle::OnMouseMove
.
void vtkCustomStyle::OnMouseMove()
{
if( m_Picked && m_OperateOnCube )
{
//Log( IInfo, "works" );
int *eventPos = m_Interator->GetEventPosition();
int *lastEventPos = m_Interator->GetLastEventPosition();
m_Renderer->SetDisplayPoint( eventPos[0], eventPos[1], 0 );
m_Renderer->DisplayToWorld();
double eventWorldPt[4];
m_Renderer->GetWorldPoint( eventWorldPt );
PointStruct eventWPos( eventWorldPt[0], eventWorldPt[1], eventWorldPt[2] );
m_Renderer->SetDisplayPoint( lastEventPos[0], lastEventPos[1], 0 );
m_Renderer->DisplayToWorld();
double lastEventWorldPt[4];
m_Renderer->GetWorldPoint( lastEventWorldPt );
PointStruct lastEventWPos( lastEventWorldPt[0], lastEventWorldPt[1], lastEventWorldPt[2] );
PointStruct moveVec = eventWPos - lastEventWPos;
vtkSmartPointer<vtkTransform> trans0 = vtkSmartPointer<vtkTransform>::New();
trans0->Translate( moveVec.point );
trans0->Update();
trans0->Concatenate( m_CubeActor->GetUserTransform() );
trans0->Update();
m_CubeActor->SetUserTransform( trans0 );
vtkPolyData *toothMesh = (vtkPolyData *)m_QuadricActor->GetMapper()->GetInput();
vtkSmartPointer<vtkPoints> sourcePts = vtkSmartPointer<vtkPoints>::New();
vtkSmartPointer<vtkPoints> targetPts = vtkSmartPointer<vtkPoints>::New();
for( int i = 0; i < m_BottomPoints->GetNumberOfPoints(); ++i )
{
PointStruct pt;
m_BottomPoints->GetPoint( i, pt.point );
trans0->TransformPoint( pt.point, pt.point );
PointStruct ptOnSurface = GetProjectedPtOnSurface( pt, toothMesh );
sourcePts->InsertNextPoint( pt.point );
targetPts->InsertNextPoint( ptOnSurface.point );
}
vtkSmartPointer<vtkLandmarkTransform> landmarkTrans = vtkSmartPointer<vtkLandmarkTransform>::New();
landmarkTrans->SetSourceLandmarks( sourcePts );
landmarkTrans->SetTargetLandmarks( targetPts );
landmarkTrans->SetModeToRigidBody();
landmarkTrans->Update();
vtkSmartPointer<vtkTransform> finalTrans = vtkSmartPointer<vtkTransform>::New();
finalTrans->Concatenate( landmarkTrans );
finalTrans->Concatenate( trans0 );
finalTrans->Update();
m_CubeActor->SetUserTransform( finalTrans );
m_RenderWindow->Render();
}
else
{
vtkInteractorStyleTrackballCamera::OnMouseMove();
}
}
You can read all source code for the branch touchCube
of my project which had been upload to GitHub, touchMoving
If you switch to the branch master, please rebuild the project and you will move an attachment on the tooth’s surface.