The example project was uploaded to GitHub: https://github.com/theArcticOcean/SimpleTools/tree/master/godotGame/NumberGameIn3D
Texture
Add texture for a mesh.
Create spatial material and set albedo texture for the object.
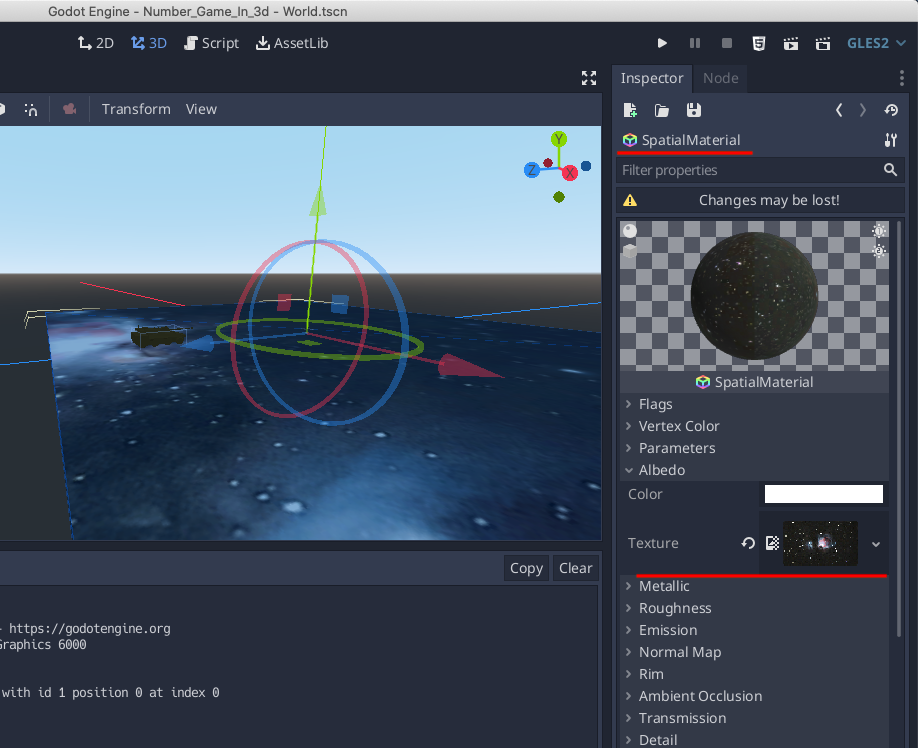
Grid Map
Create a grid map.
Create mesh object firstly, and Scene – Convert to – MeshLibrary
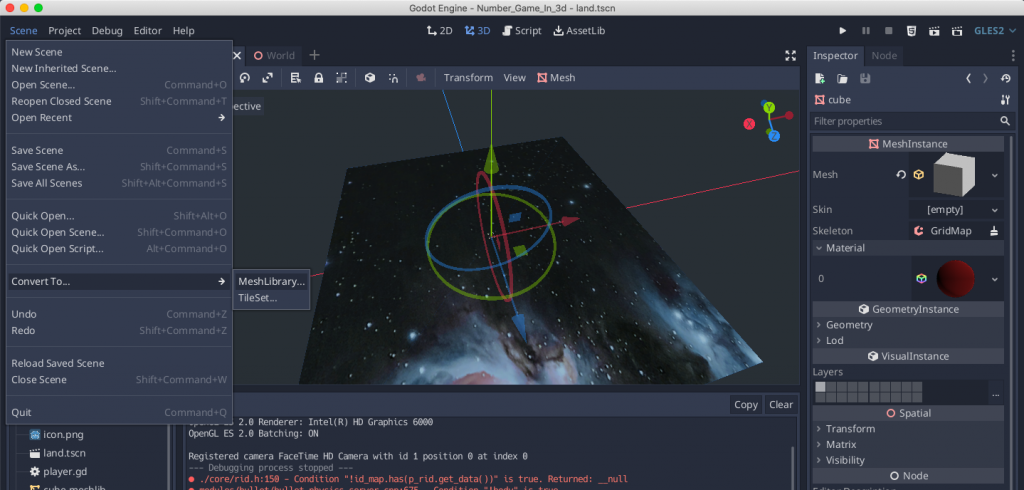
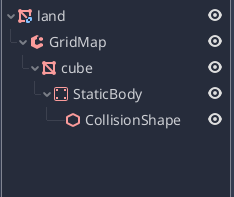
Then we can use left button press move event to create cube continuously.
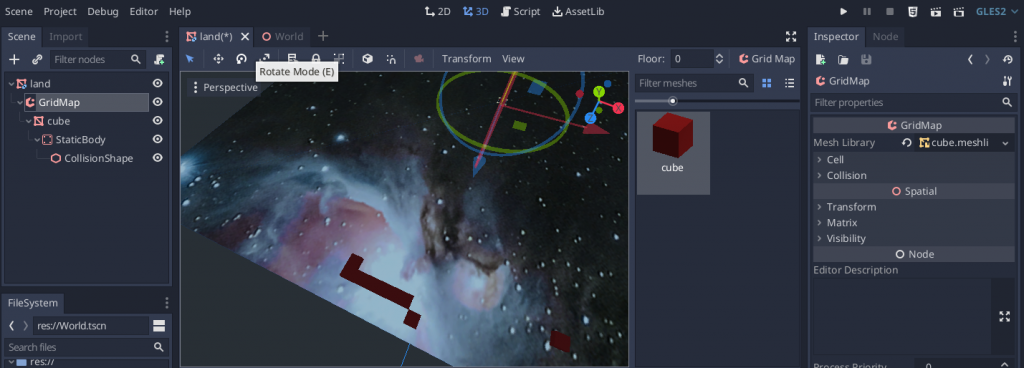
Movement
Control movements and make the camera follow the translated tank.
player.gd :
extends KinematicBody
var gravity= -ProjectSettings.get_setting( "physics/3d/default_gravity" )*2
const SPEED = 7
const ACCELERATION = 3
const DE_ACCELERATION = 5
var velocity = Vector3(0, 0, 0)
var cam
# Called when the node enters the scene tree for the first time.
func _ready():
cam = $target/Camera
func _physics_process(delta):
var cameraTrans:Transform = $target/Camera.get_global_transform()
var vec = Vector3(0, 0, 0)
vec.x = Input.get_action_strength( "move_right" ) - Input.get_action_strength( "move_left" )
vec.z = Input.get_action_strength("move_down") - Input.get_action_strength("move_up")
var YZMatrix = cameraTrans.basis.rotated( cameraTrans.basis.x, cameraTrans.basis.get_euler().x )
vec = YZMatrix.xform( vec )
if vec.length() > 0:
vec /= vec.length()
velocity.y += delta*gravity
"""var tmp = vec * SPEED
velocity.x = tmp.x
velocity.z = tmp.z"""
# avoid change too fast, create smooth effect.
var horizontalVec = velocity
horizontalVec.y = 0
print( horizontalVec )
var acceleration
if vec.dot( horizontalVec ) > 0:
acceleration = ACCELERATION
else:
acceleration = DE_ACCELERATION
var targetVec = vec * SPEED
horizontalVec = horizontalVec.linear_interpolate( targetVec, acceleration * delta )
velocity.x = horizontalVec.x
velocity.z = horizontalVec.z
velocity = move_and_slide( velocity, Vector3.UP )
if Input.is_action_pressed("jump"):
velocity.y -= delta * gravity * 3
if Input.is_action_pressed("turn_head"):
rotate_y( PI/180 )
cam.rotate_y( PI/180 )
Camera.gd:
extends Camera
export var min_distance = 5
export var max_distance = 10
export var angle_v_adjust = 0.0
var max_height = 2.0
var min_height = 0
var last_player_pos
var player
# Called when the node enters the scene tree for the first time.
func _ready():
player = get_parent().get_parent()
set_as_toplevel( true )
last_player_pos = player.global_transform.origin
func _physics_process(delta):
var target_pos: Vector3 = player.global_transform.origin
var camera_pos: Vector3 = global_transform.origin
var vec = target_pos - last_player_pos
global_transform.origin += vec
last_player_pos = target_pos
#look_at_from_position( camera_pos, target_pos, Vector3.UP ) #Vector3.UP )
Rotate
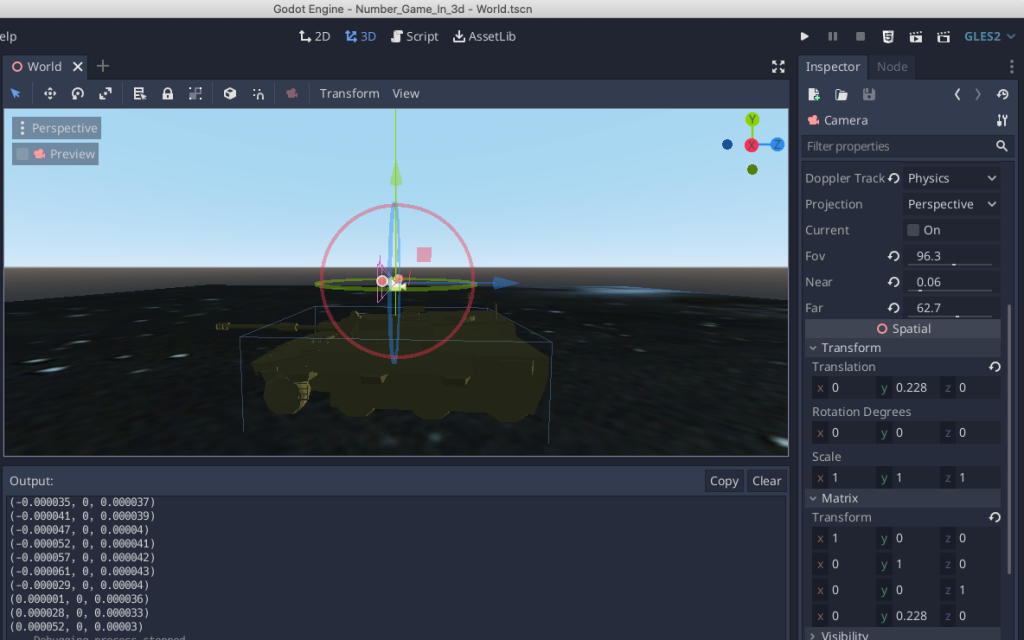
Rotate the tank and camera when moving.
func _physics_process(delta):
if Input.is_action_pressed("turn_head"):
if Input.is_action_pressed( "move_left" ):
rotate_y( PI/180 )
cam.rotate_y( PI/180 )
elif Input.is_action_pressed( "move_right" ):
rotate_y( -PI/180 )
cam.rotate_y( -PI/180 )
return
Speed up the moving.
var targetVec = vec * SPEED
if Input.is_action_pressed("speed_up"):
targetVec = targetVec * 3
[…] article is relevant to my last post, Godot: Texture, Grid Map, Move And Rotate. I want to create a 3D game by Godot, here are some notes about it. All files in the project are […]