I found a strange result about transform computation in my project. I create a new transform B by A, then use A to deep copy B and print it. There is a different matrix if I use shallow copy for A.
Turn on the Warning Display setting for vtkTransform object. I found that it would fail in the process DeepCopy.
#include <vtkTransform.h>
#define vtkSPtr vtkSmartPointer
#define vtkSPtrNew(Var, Type) vtkSPtr<Type> Var = vtkSPtr<Type>::New();
using namespace std;
int main()
{
vtkSPtrNew( trans1, vtkTransform );
trans1->Translate( 1, 0, 1 );
trans1->Scale( 1.5, 1.5, 1.5 );
trans1->Update();
vtkSPtrNew( trans2, vtkTransform );
trans2->RotateX( 50 );
trans2->Update();
vtkSPtrNew( trans3, vtkTransform );
trans3->Concatenate( trans2 );
trans3->Concatenate( trans1 );
trans3->Update();
trans2->GlobalWarningDisplayOn();
trans3->GlobalWarningDisplayOn();
trans3->GetMatrix()->PrintSelf( std::cout, vtkIndent() );
trans2->DeepCopy( trans3 );
trans2->Update();
trans2->GetMatrix()->PrintSelf( std::cout, vtkIndent() );
return 0;
}
Because trans3 will do CircuitCheck for trans2 before copying. The flag DependsOnInverse is true due to the relationship between trans2 and trans3.
The complete functions reference looks like the following code snippet.
void vtkAbstractTransform::DeepCopy(vtkAbstractTransform* transform)
//...
if (transform->CircuitCheck(this))
{
vtkErrorMacro("DeepCopy: this would create a circular reference.");
return;
}
void vtkTransform::InternalUpdate()
<=
vtkAbstractTransform* vtkTransformConcatenation::GetTransform(int i)
<=
vtkAbstractTransform* vtkAbstractTransform::GetInverse()
<=
void vtkAbstractTransform::SetInverse(vtkAbstractTransform* transform)
<=
this->DependsOnInverse = (transform != nullptr);
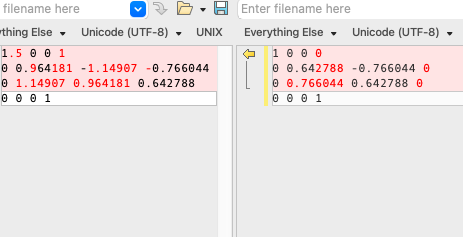