I want to cut the cone by two planes to remove the right-top part just as the following image.
Rewrite vtkClipPolyData
We can add multible clip functions for vtkClipPolyData to make it works.
#include <iostream>
#include <vtkSmartPointer.h>
#include <vtkSphereSource.h>
#include <vtkActor.h>
#include <vtkConeSource.h>
#include <vtkRenderer.h>
#include <vtkRenderWindow.h>
#include <vtkPolyDataMapper.h>
#include <vtkPlaneCollection.h>
#include <vtkClipClosedSurface.h>
#include <vtkRenderWindowInteractor.h>
#include <vtkClipPolyData.h>
#include <vtkImplicitBoolean.h>
#include <vtkPlanes.h>
#include <vtkDataArray.h>
#include <vtkDoubleArray.h>
#include <vtkProperty.h>
#include <vtkPolyLine.h>
#include "multiClipPolyData.h"
#include "point.hpp"
#include "PolydataMethods.h"
using namespace std;
#define vtkSPtr vtkSmartPointer
#define vtkSPtrNew(Var, Type) vtkSPtr<Type> Var = vtkSPtr<Type>::New();
int main()
{
vtkSPtrNew( cone, vtkConeSource );
cone->Update();
vtkSPtrNew( originData, vtkPolyData );
originData->DeepCopy( cone->GetOutput() );
CPolydataMethods::IncreaseDensity( originData, 0.1 );
vtkSPtrNew( cutPlane0, vtkPlane );
cutPlane0->SetOrigin( 0, 0, 0 );
cutPlane0->SetNormal( -1, 0, 0 );
vtkSPtrNew( cutPlane1, vtkPlane );
cutPlane1->SetOrigin( 0, 0, 0 );
cutPlane1->SetNormal( 0, -1, 0 );
vtkSPtrNew( clipper, multiClipPolyData );
clipper->SetInputData( originData );
clipper->AddClipFunction( cutPlane0 );
clipper->AddClipFunction( cutPlane1 );
clipper->Update();
vtkSPtrNew( mapper, vtkPolyDataMapper );
mapper->SetInputConnection( clipper->GetOutputPort() );
vtkSPtrNew( actor, vtkActor );
actor->SetMapper( mapper );
vtkSPtrNew( mapper0, vtkPolyDataMapper );
mapper0->SetInputData( originData );
vtkSPtrNew( actor0, vtkActor );
actor0->SetMapper( mapper0 );
actor0->GetProperty()->SetColor( 1, 0, 1 );
vtkSPtrNew( renderer, vtkRenderer );
renderer->AddActor(actor);
//renderer->AddActor(actor0);
renderer->SetBackground( 0, 0, 0 );
vtkSPtrNew( renderWindow, vtkRenderWindow );
renderWindow->AddRenderer( renderer );
vtkSPtrNew( renderWindowInteractor, vtkRenderWindowInteractor );
renderWindowInteractor->SetRenderWindow( renderWindow );
renderer->ResetCamera();
renderWindow->Render();
renderWindowInteractor->Start();
return 0;
}
The files about the class multiClipPolyData had been push to GitHub, cut mesh by multiple planes.
Use vtkPlanes
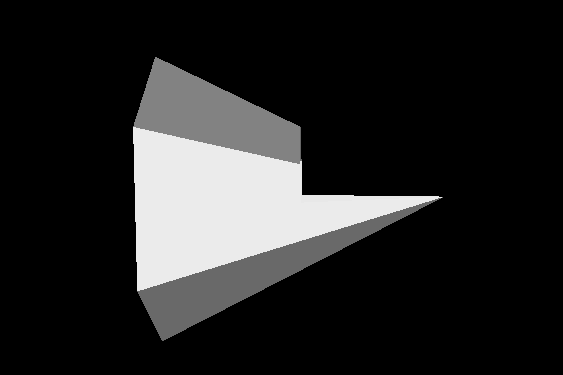
#include <iostream>
#include <vtkSmartPointer.h>
#include <vtkSphereSource.h>
#include <vtkActor.h>
#include <vtkConeSource.h>
#include <vtkRenderer.h>
#include <vtkRenderWindow.h>
#include <vtkPolyDataMapper.h>
#include <vtkPlaneCollection.h>
#include <vtkClipClosedSurface.h>
#include <vtkRenderWindowInteractor.h>
#include <vtkClipPolyData.h>
#include <vtkImplicitBoolean.h>
#include <vtkPlanes.h>
#include <vtkDataArray.h>
#include <vtkDoubleArray.h>
#include <vtkProperty.h>
#include <vtkPolyLine.h>
#include "multiClipPolyData.h"
#include "point.hpp"
#include "PolydataMethods.h"
using namespace std;
#define vtkSPtr vtkSmartPointer
#define vtkSPtrNew(Var, Type) vtkSPtr<Type> Var = vtkSPtr<Type>::New();
int main()
{
vtkSPtrNew( cone, vtkConeSource );
cone->Update();
vtkSPtrNew( originData, vtkPolyData );
originData->DeepCopy( cone->GetOutput() );
CPolydataMethods::IncreaseDensity( originData, 0.1 );
vtkSPtrNew( cutPlanes, vtkPlanes );
vtkSPtrNew( cutPts, vtkPoints );
cutPts->InsertNextPoint( 0, 0, 0 ); // set origin of cut plane
cutPts->InsertNextPoint( 0.5, 0, 0 );
vtkSPtrNew( normals, vtkDoubleArray );
normals->SetNumberOfComponents( 3 );
normals->InsertTuple3( 0, -1, 0, 0 ); // set normal of cut plane
normals->InsertTuple3( 1, 0, -1, 0 );
cutPlanes->SetPoints( cutPts );
cutPlanes->SetNormals( normals );
vtkSPtrNew( clipper, vtkClipPolyData );
clipper->SetInputData( originData );
clipper->SetClipFunction( cutPlanes );
clipper->Update();
vtkSPtrNew( mapper, vtkPolyDataMapper );
mapper->SetInputConnection( clipper->GetOutputPort() );
vtkSPtrNew( actor, vtkActor );
actor->SetMapper( mapper );
vtkSPtrNew( mapper0, vtkPolyDataMapper );
mapper0->SetInputData( originData );
vtkSPtrNew( actor0, vtkActor );
actor0->SetMapper( mapper0 );
actor0->GetProperty()->SetColor( 1, 0, 1 );
vtkSPtrNew( renderer, vtkRenderer );
renderer->AddActor(actor);
//renderer->AddActor(actor0);
renderer->SetBackground( 0, 0, 0 );
vtkSPtrNew( renderWindow, vtkRenderWindow );
renderWindow->AddRenderer( renderer );
vtkSPtrNew( renderWindowInteractor, vtkRenderWindowInteractor );
renderWindowInteractor->SetRenderWindow( renderWindow );
renderer->ResetCamera();
renderWindow->Render();
renderWindowInteractor->Start();
return 0;
}
Use vtkImplicitBoolean
#include <iostream>
#include <vtkSmartPointer.h>
#include <vtkSphereSource.h>
#include <vtkActor.h>
#include <vtkConeSource.h>
#include <vtkRenderer.h>
#include <vtkRenderWindow.h>
#include <vtkPolyDataMapper.h>
#include <vtkPlaneCollection.h>
#include <vtkClipClosedSurface.h>
#include <vtkRenderWindowInteractor.h>
#include <vtkClipPolyData.h>
#include <vtkImplicitBoolean.h>
#include <vtkPlanes.h>
#include <vtkDataArray.h>
#include <vtkDoubleArray.h>
#include <vtkProperty.h>
#include <vtkPolyLine.h>
#include <vtkImplicitBoolean.h>
#include "multiClipPolyData.h"
#include "point.hpp"
#include "PolydataMethods.h"
using namespace std;
#define vtkSPtr vtkSmartPointer
#define vtkSPtrNew(Var, Type) vtkSPtr<Type> Var = vtkSPtr<Type>::New();
int main()
{
vtkSPtrNew( cone, vtkConeSource );
cone->Update();
vtkSPtrNew( originData, vtkPolyData );
originData->DeepCopy( cone->GetOutput() );
CPolydataMethods::IncreaseDensity( originData, 0.1 );
vtkSPtrNew( cutPlane0, vtkPlane );
cutPlane0->SetNormal( -1, 0, 0 );
cutPlane0->SetOrigin( 0, 0, 0 );
vtkSPtrNew( cutPlane1, vtkPlane );
cutPlane1->SetNormal( 0, -1, 0 );
cutPlane1->SetOrigin( 0.5, 0, 0 );
vtkSPtrNew( boolean, vtkImplicitBoolean );
boolean->AddFunction( cutPlane0 );
boolean->AddFunction( cutPlane1 );
//boolean->SetOperationTypeToUnion();
//boolean->SetOperationTypeToUnionOfMagnitudes();
boolean->SetOperationTypeToIntersection();
vtkSPtrNew( clipper, vtkClipPolyData );
clipper->SetInputData( originData );
clipper->SetClipFunction( boolean );
clipper->Update();
vtkSPtrNew( mapper, vtkPolyDataMapper );
mapper->SetInputConnection( clipper->GetOutputPort() );
vtkSPtrNew( actor, vtkActor );
actor->SetMapper( mapper );
vtkSPtrNew( mapper0, vtkPolyDataMapper );
mapper0->SetInputData( originData );
vtkSPtrNew( actor0, vtkActor );
actor0->SetMapper( mapper0 );
actor0->GetProperty()->SetColor( 1, 0, 1 );
vtkSPtrNew( renderer, vtkRenderer );
renderer->AddActor(actor);
//renderer->AddActor(actor0);
renderer->SetBackground( 0, 0, 0 );
vtkSPtrNew( renderWindow, vtkRenderWindow );
renderWindow->AddRenderer( renderer );
vtkSPtrNew( renderWindowInteractor, vtkRenderWindowInteractor );
renderWindowInteractor->SetRenderWindow( renderWindow );
renderer->ResetCamera();
renderWindow->Render();
renderWindowInteractor->Start();
return 0;
}
vtkClipClosedSurface can be used to generate clipped polydata which is closed.
Usage:
vSPNew( cutPlane0, vtkPlane );
cutPlane0->SetOrigin( cutPtOnTooth[0].point );
cutPlane0->SetNormal( normal.point );
vSPNew( cutPlane0Collect, vtkPlaneCollection );
cutPlane0Collect->AddItem( cutPlane0 );
vSPNew( clipClosed0, vtkClipClosedSurface );
clipClosed0->SetInputData( modelData);
clipClosed0->SetClippingPlanes( cutPlane0Collect );
clipClosed0->Update();