Create a simple cone and plane.
#include <iostream>
#include <vtkSmartPointer.h>
#include <vtkSphereSource.h>
#include <vtkActor.h>
#include <vtkConeSource.h>
#include <vtkRenderer.h>
#include <vtkRenderWindow.h>
#include <vtkPolyDataMapper.h>
#include <vtkProperty.h>
#include <vtkRenderWindowInteractor.h>
#include <vtkLight.h>
#include <vtkCamera.h>
#include <vtkOBBTree.h>
#include <vtkActor2D.h>
#include <vtkMath.h>
#include <vtkTransform.h>
#include <vtkTransformFilter.h>
#include <vtkMatrix4x4.h>
#include <vtkInteractorObserver.h>
#include <vtkPolyDataNormals.h>
#include <vtkOutlineFilter.h>
#include <vtkPlaneSource.h>
#include "tool.h"
using namespace std;
int main()
{
setbuf( stdout, nullptr );
vtkSmartPointer<vtkPlaneSource> planeSource =
vtkSmartPointer<vtkPlaneSource>::New();
planeSource->SetCenter( -0.5, 0, 0 );
planeSource->SetNormal( 1, 0, 0 );
planeSource->Update();
vtkSmartPointer<vtkPolyDataMapper> planeMapper =
vtkSmartPointer<vtkPolyDataMapper>::New();
planeMapper->SetInputData( planeSource->GetOutput() );
planeMapper->Update();
vtkSmartPointer<vtkActor> planeActor =
vtkSmartPointer<vtkActor>::New();
planeActor->SetMapper( planeMapper );
planeActor->GetProperty()->SetColor( 0, 1, 0 );
vtkSmartPointer<vtkConeSource> cone =
vtkSmartPointer<vtkConeSource>::New();
//cone->SetDirection( 1, 1, 0 );
cone->Update();
PointStruct center, max, mid, min, size;
vtkSmartPointer<vtkOBBTree> obbTree =
vtkSmartPointer<vtkOBBTree>::New();
obbTree->ComputeOBB( cone->GetOutput(),
center.point,
max.point,
mid.point,
min.point,
size.point );
vtkMath::Normalize( center.point );
vtkMath::Normalize( max.point );
vtkMath::Normalize( mid.point );
vtkMath::Normalize( min.point );
cout << "center: " << center;
cout << "max: " << max;
cout << "mid: " << mid;
cout << "min: " << min;
vtkSmartPointer<vtkPolyDataMapper> mapper =
vtkSmartPointer<vtkPolyDataMapper>::New();
mapper->SetInputData( cone->GetOutput() );
vtkSmartPointer<vtkActor> actor =
vtkSmartPointer<vtkActor>::New();
actor->SetMapper( mapper );
vtkSmartPointer<vtkRenderer> renderer =
vtkSmartPointer<vtkRenderer>::New();
renderer->AddActor(actor);
renderer->AddActor(planeActor);
renderer->SetBackground( 0, 0, 0 );
vtkSmartPointer<vtkRenderWindow> renderWindow =
vtkSmartPointer<vtkRenderWindow>::New();
renderWindow->AddRenderer( renderer );
vtkSmartPointer<vtkRenderWindowInteractor> renderWindowInteractor =
vtkSmartPointer<vtkRenderWindowInteractor>::New();
renderWindowInteractor->GetInteractorStyle()->SetCurrentRenderer( renderer );
renderWindowInteractor->SetRenderWindow( renderWindow );
renderer->ResetCamera();
renderWindow->Render();
renderWindowInteractor->Start();
return 0;
}
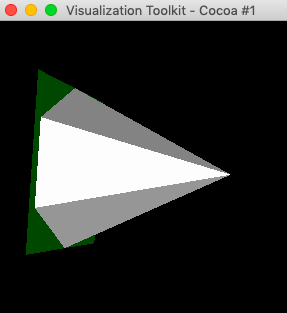
Rotate cone, make cone->SetDirection( 1, 1, 0 );
works.
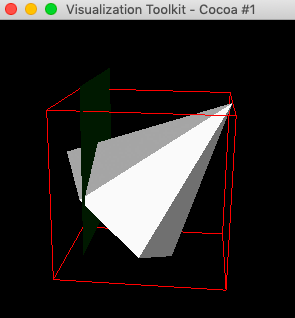
Use bounding box point to set plane at left side.
double boundingBox[6] = { 0 };
cone->GetOutput()->GetBounds( boundingBox );
PointStruct pt0( boundingBox[0], boundingBox[3], boundingBox[5] );
PointStruct pt1( boundingBox[0], boundingBox[3], boundingBox[4] );
PointStruct pt2( boundingBox[0], boundingBox[2], boundingBox[4] );
PointStruct centerPt = pt0 + pt2;
centerPt /= 2;
vtkSmartPointer<vtkPlaneSource> planeSource =
vtkSmartPointer<vtkPlaneSource>::New();
//planeSource->SetPoint1( (pt1 - pt0).point );
//planeSource->SetPoint2( (pt2 - pt0).point );
planeSource->SetCenter( centerPt.point );
planeSource->SetNormal( 1, 0, 0 );
planeSource->Update();
vtkSmartPointer<vtkPolyDataMapper> planeMapper =
vtkSmartPointer<vtkPolyDataMapper>::New();
planeMapper->SetInputData( planeSource->GetOutput() );
planeMapper->Update();
vtkSmartPointer<vtkActor> planeActor =
vtkSmartPointer<vtkActor>::New();
planeActor->SetMapper( planeMapper );
planeActor->GetProperty()->SetColor( 0, 1, 0 );
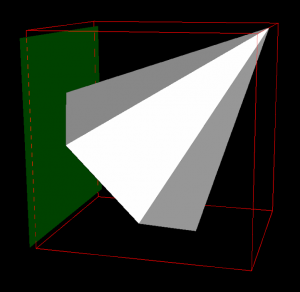
How to find the intersections point between plane and cone?
vtkCutter can help us to find all intersections.
// Create cutter
vtkSmartPointer<vtkCutter> cutter =
vtkSmartPointer<vtkCutter>::New();
cutter->SetCutFunction( planeFunction );
cutter->SetInputData( cone->GetOutput() );
cutter->Update();
cout << "Cutter GetNumberOfPoints: " << cutter->GetOutput()->GetNumberOfPoints() << endl;
vtkSmartPointer<vtkPolyDataMapper> cutterMapper =
vtkSmartPointer<vtkPolyDataMapper>::New();
cutterMapper->SetInputData( cutter->GetOutput() );
vtkSmartPointer<vtkActor> cutterActor =
vtkSmartPointer<vtkActor>::New();
cutterActor->SetMapper( cutterMapper );
cutterActor->GetProperty()->SetColor( 1, 0, 0 );
```
If we put a plane on the bounding box, it can’t find the intersection.
So we should move the plane a little closer.
```cpp
vtkSmartPointer<vtkPlaneSource> planeSource =
vtkSmartPointer<vtkPlaneSource>::New();
centerPt[0] = centerPt[0] + 0.01; //move it
planeSource->SetCenter( centerPt.point );
planeSource->SetNormal( 1, 0, 0 );
planeSource->Update();
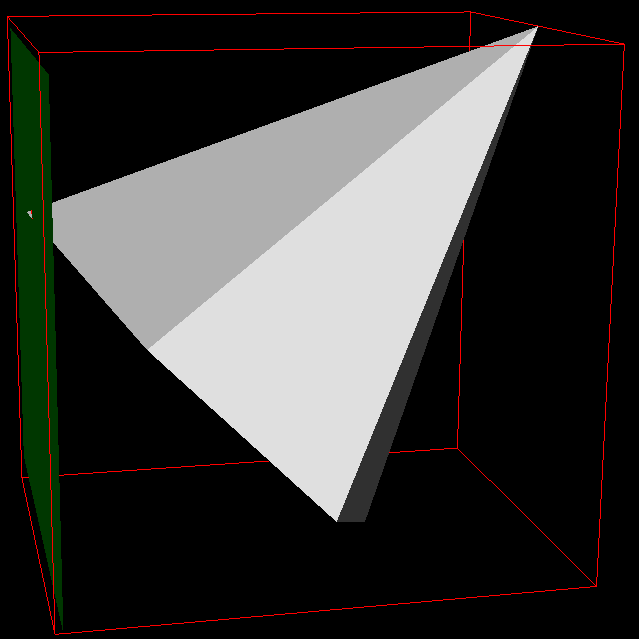
Output:
Cutter GetNumberOfPoints: 4
Look it at wireframe representation.
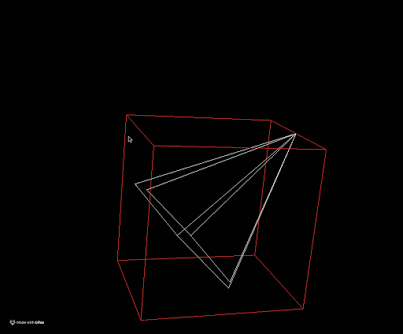