vtkImageActor
vtkImageActor is used to display picture in 3D render window.
By changing the interactor to a vtkInteractorStyleImage you can limit rotations so that user can operate the 3D render window as a 2D image viewer.
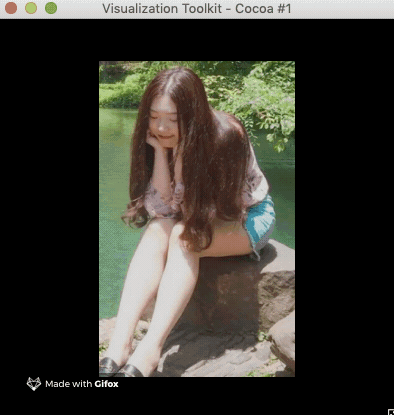
import vtk
fileName = "/Users/weiyang/Desktop/Untitled.png"
reader = vtk.vtkPNGReader()
reader.SetFileName( fileName )
reader.Update()
actor = vtk.vtkImageActor()
actor.SetInputData( reader.GetOutput() )
ren = vtk.vtkRenderer()
ren.AddActor( actor )
renWin = vtk.vtkRenderWindow()
renWin.AddRenderer( ren )
iren = vtk.vtkRenderWindowInteractor()
iren.SetRenderWindow( renWin )
iren.Initialize()
iStyle = vtk.vtkInteractorStyleImage()
iren.SetInteractorStyle( iStyle )
ren.AddActor( actor )
renWin.SetSize( 400, 400 )
renWin.Render()
ren.ResetCameraClippingRange()
renWin.Render()
iren.Start()
vtkImagePlaneWidget
vtkImagePlaneWidget can change position and orientation manually. User can reslice data with different methods such as VTK_NEAREST_RESLICE
, VTK_LINEAR_RESLICE
, and VTK_CUBIC_RESLICE
.
Add the following code before AddActor in the above example.
planeWidgetX = vtk.vtkImagePlaneWidget()
planeWidgetX.SetInteractor( iren )
planeWidgetX.RestrictPlaneToVolumeOn()
planeWidgetX.SetResliceInterpolateToNearestNeighbour()
planeWidgetX.SetInputData( reader.GetOutput() )
planeWidgetX.SetPlaneOrientationToZAxes()
planeWidgetX.SetSliceIndex( 0 )
planeWidgetX.DisplayTextOn()
planeWidgetX.SetResliceInterpolateToNearestNeighbour()
planeWidgetX.On()
An interesting thing to notice is that planeWidgetX need not be added to the renderer as vtkActor object because it’s a widget.
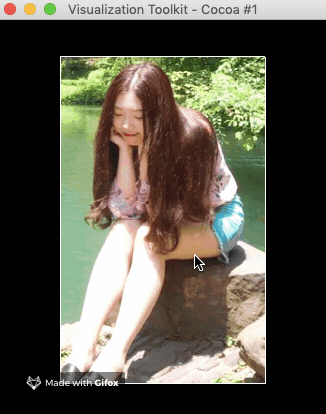
void vtkImagePlaneWidget::ProcessEvents(vtkObject* vtkNotUsed(object),
unsigned long event,
void* clientdata,
void* vtkNotUsed(calldata))
void vtkImagePlaneWidget::OnLeftButtonDown()
void vtkImagePlaneWidget::StartCursor()
void vtkImagePlaneWidget::ManageTextDisplay()
The fourth value in the coordinate result on the gif is CurrentImageValue.
The comment of int vtkImagePlaneWidget::UpdateContinuousCursor(double *q)
has mentioned that this->CurrentImageValue = outPD->GetScalars()->GetTuple1(0);
, so it has relationship with scalar.