I have used CGAL to do simple tasks, the related article: Simple Demos About Using CGAL. But those tasks work in header mode, I want to build CGAL libraries with source code and install them.
Create a folder named build and a script build.sh in it.
build.sh:
cmake ../ -G "Unix Makefiles" \
-DCGAL_HEADER_ONLY=OFF \
-DCMAKE_BUILD_TYPE=Release \
-DQt5_DIR:PATH=/usr/local/Qt-5.15.1/lib/cmake/Qt5 \
-DVTK_SMP_IMPLEMENTATION_TYPE:STRING=TBB \
-DTBB_INCLUDE_DIR:PATH=/Users/weiyang/Downloads/tbb/include \
-DTBB_LIBRARY_DEBUG:FILEPATH=/Users/weiyang/Downloads/tbb/build/macos_intel64_clang_cc11.0.0_os10.15_debug/libtbb_debug.dylib \
-DTBB_LIBRARY_RELEASE:FILEPATH=/Users/weiyang/Downloads/tbb/build/macos_intel64_clang_cc11.0.0_os10.15_release/libtbb.dylib \
-DTBB_MALLOC_INCLUDE_DIR:PATH=/Users/weiyang/Downloads/tbb/include \
-DTBB_MALLOC_LIBRARY_DEBUG:FILEPATH=/Users/weiyang/Downloads/tbb/build/macos_intel64_clang_cc11.0.0_os10.15_debug/libtbbmalloc_debug.dylib \
-DTBB_MALLOC_LIBRARY_RELEASE:FILEPATH=/Users/weiyang/Downloads/tbb/build/macos_intel64_clang_cc11.0.0_os10.15_release/libtbbmalloc.dylib \
-DTBB_MALLOC_PROXY_INCLUDE_DIR:PATH=/Users/weiyang/Downloads/tbb/include \
-DTBB_MALLOC_PROXY_LIBRARY_DEBUG:FILEPATH=/Users/weiyang/Downloads/tbb/build/macos_intel64_clang_cc11.0.0_os10.15_debug/libtbbmalloc_proxy_debug.dylib \
-DTBB_MALLOC_PROXY_LIBRARY_RELEASE:FILEPATH=/Users/weiyang/Downloads/tbb/build/macos_intel64_clang_cc11.0.0_os10.15_release/libtbbmalloc_proxy.dylib \
-DZLIB_LIBRARY_RELEASE:FILEPATH=/usr/local/opt/zlib/lib/libz.dylib \
-DZLIB_INCLUDE_DIR:FILEPATH=/usr/local/opt/zlib/include
Go to the folder path and run the script.
Bash build.sh.
...
-- Skip whitelisted header "CGAL/CGAL_Ipelet_base.h".
-- Skip whitelisted header "CGAL/Linear_cell_complex_constructors.h".
-- Skip LEDA header "CGAL/leda_bigfloat.h" because LEDA_FOUND is false.
-- Skip LEDA header "CGAL/leda_bigfloat_interval.h" because LEDA_FOUND is false.
-- Skip LEDA header "CGAL/leda_coercion_traits.h" because LEDA_FOUND is false.
-- Skip LEDA header "CGAL/leda_integer.h" because LEDA_FOUND is false.
-- Skip LEDA header "CGAL/leda_rational.h" because LEDA_FOUND is false.
-- Skip LEDA header "CGAL/leda_real.h" because LEDA_FOUND is false.
-- Skip whitelisted header "CGAL/GLPK_mixed_integer_program_traits.h".
-- Skip whitelisted header "CGAL/SCIP_mixed_integer_program_traits.h".
You can now check the headers with the target `check_headers`
and the package dependencies with the target `packages_dependencies`.
Results are in the `package_info/` sub-directory of the build directory:
- package_info/<package>/dependencies
- package_info/<package>/included_headers
- package_info/<package>/check_headers/ (error messages from the headers checks)
== Setting header checking (DONE) ==
-- Configuring done
-- Generating done
Then we can build the project and install libraries.
Create a matrix and do transpose operation.
CMakeLists.txt:
cmake_minimum_required(VERSION 3.1...3.15)
project(CGAL_Test)
find_package(CGAL REQUIRED)
set(CMAKE_CXX_STANDARD 14)
# Link with Boost.ProgramOptions (optional)
find_package(Boost QUIET COMPONENTS program_options)
if(Boost_PROGRAM_OPTIONS_FOUND)
if(TARGET Boost::program_options)
set(Boost_PROGRAM_OPTIONS_LIBRARY Boost::program_options)
endif()
if(CGAL_AUTO_LINK_ENABLED)
message(STATUS "Boost.ProgramOptions library: found")
else()
message(
STATUS "Boost.ProgramOptions library: ${Boost_PROGRAM_OPTIONS_LIBRARY}")
endif()
add_definitions("-DCGAL_USE_BOOST_PROGRAM_OPTIONS")
list(APPEND CGAL_3RD_PARTY_LIBRARIES ${Boost_PROGRAM_OPTIONS_LIBRARY})
endif()
# Find Eigen3 (requires 3.1.0 or greater)
find_package(Eigen3 3.1.0)
include(CGAL_Eigen_support)
create_single_source_cgal_program( "main.cpp" )
target_link_libraries(main PUBLIC CGAL::Eigen_support)
main.cpp
#include <CGAL/Linear_algebraHd.h>
#include <CGAL/Linear_algebraCd.h>
#include <iostream>
using namespace std;
using namespace CGAL::Linear_Algebra;
typedef CGAL::Linear_algebraCd<double> LA;
typedef LA::Matrix Matrix;
typedef LA::Vector Vector;
int main()
{
std::vector<Vector> elements;
std::vector<double> tmp = { 1, 1, 2, 3 };
elements.push_back( Vector( tmp.begin(), tmp.end() ) );
tmp = { 0, 1, 0, 3 };
elements.push_back( Vector( tmp.begin(), tmp.end() ) );
tmp = { 0, 2, 5, 1 };
elements.push_back( Vector( tmp.begin(), tmp.end() ) );
tmp = { 0, 0, 10, 9};
elements.push_back( Vector( tmp.begin(), tmp.end() ) );
Matrix M( elements.begin(), elements.end() );
CGAL::set_mode( cout, CGAL::IO::PRETTY );
cout << M << endl;
auto MT = LA::transpose( M );
cout << MT << endl;
return 0;
}
Output:
LA::Matrix((4, 4 [1, 0, 0, 0,
1, 1, 2, 0,
2, 0, 5, 10,
3, 3, 1, 9,
])
LA::Matrix((4, 4 [1, 1, 2, 3,
0, 1, 0, 3,
0, 2, 5, 1,
0, 0, 10, 9,
])
We can search keywords in the include path to find the header file which we need when writing the program.
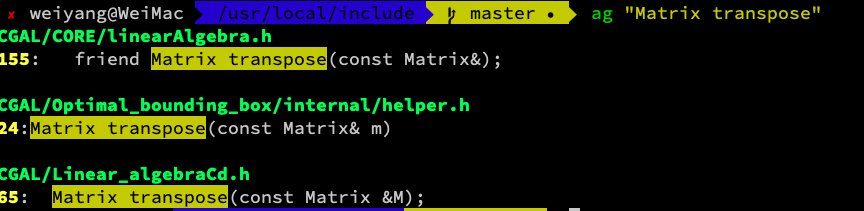