Js code call C interface synchronously, it will wait if the interface is in an infinite loop.
Write a function which has an infinite loop.
#include <stdio.h>
// export the function.
#ifdef __EMSCRIPTEN__
#include <emscripten.h>
EMSCRIPTEN_KEEPALIVE
#endif
int compute( int a, int b )
{
int ans = 1;
while( 1 )
{
};
for( int i = 0; i < b; ++i )
{
ans = ans * a;
}
return ans;
}
Here is html page
<!doctype html>
<html lang="en">
<head>
<title>Test</title>
<meta charset="utf-8"/>
</head>
<body>
<script async type="text/javascript" src="Tester.js"></script>
<button id="button" onclick="Calculate()">Calculate</button>
<script>
var instance = null;
async function fetchAndInstantiate() {
const response = await fetch("Tester.wasm");
const buffer = await response.arrayBuffer();
const obj = await WebAssembly.instantiate(buffer);
instance = obj.instance;
}
fetchAndInstantiate();
function Calculate()
{
console.log( "start" );
const ans = instance.exports.compute(2, 10);
console.log( "finish" );
console.log( ans );
}
</script>
</body>
</html>
Compile C file to wasm and JS file.
emcc Tester.c -o Tester.js
The web page will be blocked after click on the button to call C interface.
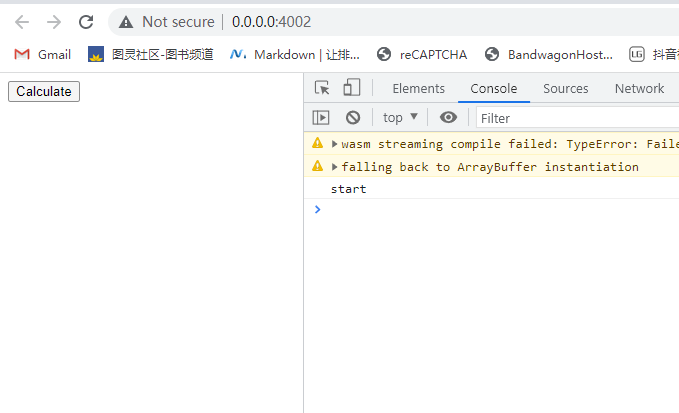
[…] synchronous for JS code call wasm exported interface as introduced in post Synchronous call between JS and C function.Asynchronous call can be implemented by js code […]