This post is based on an old project Use ITK And VTK To Show DICOM Image.
We want to add an above layer which drawed translucent red color on the gray image.
Use alpha blend algorithm to merge two different color:
origin color =
canvas color =
result color =
vtkImageData *data = imgToColors->GetOutput();
vtkInformation* outInfo = alg->GetOutputInformation(0);
int *w_ext = outInfo->Get(vtkStreamingDemandDrivenPipeline::WHOLE_EXTENT());
vtkSmartPointer<vtkImageActor> actor = vtkSmartPointer<vtkImageActor>::New();
actor->SetInputData( data );
int slice = (w_ext[4] + w_ext[5])/2;
actor->SetDisplayExtent( w_ext[0], w_ext[1], w_ext[2], w_ext[3], slice, slice );
cout << "GetNumberOfScalarComponents: " << data->GetNumberOfScalarComponents() << endl;
cout << "GetNumberOfPoints: " << data->GetNumberOfPoints() << endl;
double *range = data->GetScalarRange();
cout << "scalar range: " << range[0] << ", " << range[1] << endl;
cout << "GetScalarTypeAsString: " << data->GetScalarTypeAsString() << endl;
cout << "GetScalarSize: " << data->GetScalarSize() << endl;
unsigned char *unitScalar = (unsigned char*)( data->GetScalarPointer( slice, slice, slice ) );
cout << "unitScalar: " << unitScalar[0] << ", " << unitScalar[1] << ", " << unitScalar[2] << ", " << int(unitScalar[3]) << endl;
cout << "GetScalarComponentAsDouble: " << data->GetScalarComponentAsDouble( slice, slice, slice, 3 ) << endl;
for( int i = w_ext[0]; i < w_ext[1]; ++i )
{
for( int j = w_ext[2]; j < w_ext[3]; ++j )
{
unsigned char *unitScalar = (unsigned char*)( data->GetScalarPointer( i, j, slice ) );
cout << "unitScalar: " << int(unitScalar[0]) << ", " << int(unitScalar[1]) << ", " << int(unitScalar[2]) << ", " << int(unitScalar[3]) << endl;
double oldColor[4] = { unitScalar[0], unitScalar[1], unitScalar[2], unitScalar[3] };
double canvasColor[4] = { 255, 0, 0, 127 };
double newColor[4] = { (canvasColor[0]*canvasColor[3] + oldColor[0]*(255-canvasColor[3])) / 255.0,
(canvasColor[1]*canvasColor[3] + oldColor[1]*(255-canvasColor[3])) / 255.0,
(canvasColor[2]*canvasColor[3] + oldColor[2]*(255-canvasColor[3])) / 255.0,
255 };
data->SetScalarComponentFromDouble( i, j, slice, 0, newColor[0] );
data->SetScalarComponentFromDouble( i, j, slice, 1, newColor[1] );
data->SetScalarComponentFromDouble( i, j, slice, 2, newColor[2] );
data->SetScalarComponentFromDouble( i, j, slice, 3, newColor[3] );
}
}
vtkSmartPointer<vtkRenderer> ren = vtkSmartPointer<vtkRenderer>::New();
ren->AddActor( actor );
Result:
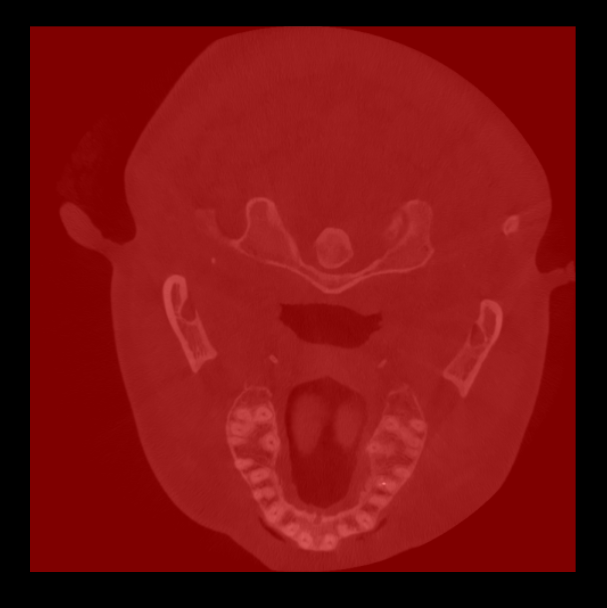