The post introduces two topics.
- how to pass
vector<int>
from javascript to the interface exported from wasm - how to get
vector<int>
object from wasm and print values one by one.
My explore was based on the old project from What will happen if we pass wrong vtkRenderer object for vtkPicker::Pick?.
Just see the important logics about our issue at the following diff file.
vector.diff:
diff --git a/learnWebAssembly/cellPickerInWrongRenderer/binding.cpp b/learnWebAssembly/cellPickerInWrongRenderer/binding.cpp
index baf24a6..41495f9 100644
--- a/learnWebAssembly/cellPickerInWrongRenderer/binding.cpp
+++ b/learnWebAssembly/cellPickerInWrongRenderer/binding.cpp
@@ -8,5 +8,9 @@ EMSCRIPTEN_BINDINGS(worker) {
.constructor()
.function("Init", &Worker::Init)
.function("Start", &Worker::Start)
+ .function("PrintVector", &Worker::PrintVector)
+ .function("GetVector", &Worker::GetVector)
;
+
+ register_vector<int>("IntVector");
}
\ No newline at end of file
diff --git a/learnWebAssembly/cellPickerInWrongRenderer/index.html b/learnWebAssembly/cellPickerInWrongRenderer/index.html
index 39800b1..ef6ec8a 100644
--- a/learnWebAssembly/cellPickerInWrongRenderer/index.html
+++ b/learnWebAssembly/cellPickerInWrongRenderer/index.html
@@ -45,6 +45,18 @@
$('button').onclick = function()
{
console.log( "onclick!" );
+ const vecObj = new Module.IntVector();
+ vecObj.push_back( 1 );
+ vecObj.push_back( 2 );
+ vecObj.push_back( 3 );
+ workerObj.PrintVector( vecObj );
+
+ const vecInWasm = workerObj.GetVector();
+ for( let i = 0; i < vecInWasm.size(); ++i )
+ {
+ const value = vecInWasm.get( i );
+ console.log( "value: ", value );
+ }
}
</script>
</body>
diff --git a/learnWebAssembly/cellPickerInWrongRenderer/worker.cpp b/learnWebAssembly/cellPickerInWrongRenderer/worker.cpp
index 1bde04f..daa7eb1 100644
--- a/learnWebAssembly/cellPickerInWrongRenderer/worker.cpp
+++ b/learnWebAssembly/cellPickerInWrongRenderer/worker.cpp
@@ -72,4 +72,18 @@ void Worker::OnLeftButtonDown()
vtkSPtr<vtkActor> actor = m_Picker->GetActor();
Log( IInfo, "actor: ", actor );
}
+}
+
+void Worker::PrintVector( std::vector<int> vec )
+{
+ for( auto value: vec )
+ {
+ Log( IInfo, "value: ", value );
+ }
+}
+
+std::vector<int> Worker::GetVector()
+{
+ std::vector<int> result = { 1, 2, 3 };
+ return result;
}
\ No newline at end of file
diff --git a/learnWebAssembly/cellPickerInWrongRenderer/worker.h b/learnWebAssembly/cellPickerInWrongRenderer/worker.h
index aa6fe1f..1bc4a54 100644
--- a/learnWebAssembly/cellPickerInWrongRenderer/worker.h
+++ b/learnWebAssembly/cellPickerInWrongRenderer/worker.h
@@ -11,6 +11,7 @@
#include <vtkRenderWindowInteractor.h>
#include <vtkPropPicker.h>
#include <vtkCellPicker.h>
+#include <vector>
#ifdef __EMSCRIPTEN__
#include "vtkSDL2OpenGLRenderWindow.h"
@@ -28,6 +29,9 @@ public:
void Init();
void Start();
void OnLeftButtonDown();
+
+ void PrintVector( std::vector<int> vec );
+ std::vector<int> GetVector();
protected:
#ifdef __EMSCRIPTEN__
vtkSPtr<vtkSDL2RenderWindowInteractor> m_RenderWindowInteractor;
Rememder use register_vector
to export vector<int>
and use it in js when declare data type of vecObj.
The result was displayed after clicking the left-bottom button on the web page.
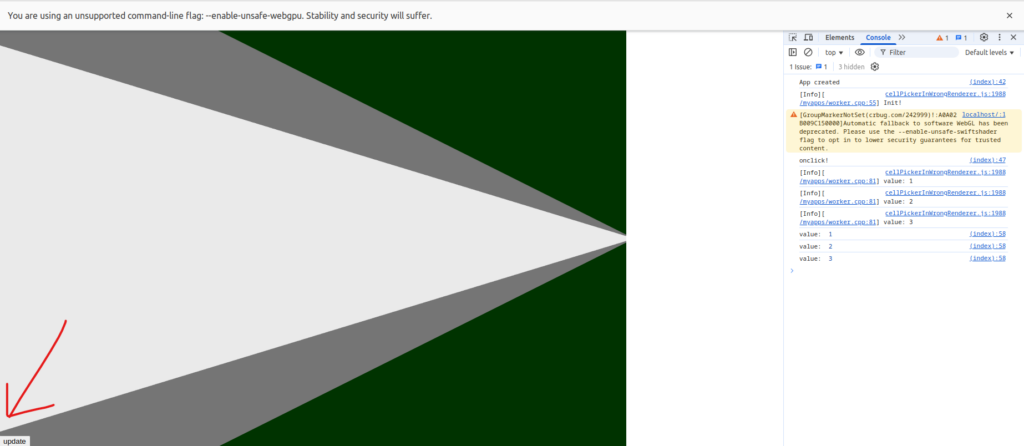
.
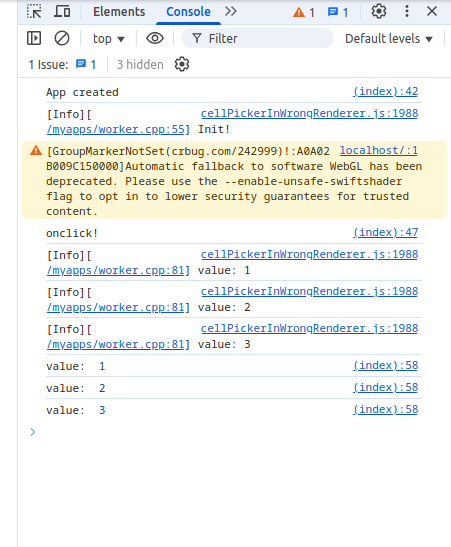
You can use the diff file directly for the old project in What will happen if we pass wrong vtkRenderer object for vtkPicker::Pick?.
git apply --check vector.diff