We have added a new feature to the 3D Model Editor. It can remove non-monifold edges and non-monifold points.
The non-monifold edges and non-monifold points are introduced at https://www.weiy.city/2022/09/3d-model-editor-find-non-manifold-edge-and-points/.
Let’s create a cube with non-monifold edges and non-monifold points by the following code.
#include <iostream>
#include <vtkSmartPointer.h>
#include <vtkTransform.h>
#include <vtkActor.h>
#include <vtkConeSource.h>
#include <vtkRenderer.h>
#include <vtkRenderWindow.h>
#include <vtkPolyDataMapper.h>
#include <vtkRenderWindowInteractor.h>
#include <vtkAxesActor.h>
#include <vtkLine.h>
#include <vtkPlane.h>
#include <vtkSTLWriter.h>
#include "point.hpp"
using namespace std;
#define vtkSPtr vtkSmartPointer
#define vtkSPtrNew(Var, Type) vtkSPtr<Type> Var = vtkSPtr<Type>::New();
int main()
{
vtkSPtrNew( points, vtkPoints );
points->InsertNextPoint( -1, 0, 1 );
points->InsertNextPoint( 1, 0, 1 );
points->InsertNextPoint( 1, 0, -1 );
points->InsertNextPoint( -1, 0, -1 );
vtkSPtrNew( plane, vtkPlane );
plane->SetOrigin( 0, 2, 0 );
plane->SetNormal( 0, -1, 0 );
// ==================== start to generate cube ====================
for( int i = 0; i < 4; ++i )
{
Point projectPt = Point( points->GetPoint( i ) );
plane->ProjectPoint( projectPt.point, projectPt.point );
points->InsertNextPoint( projectPt.point );
}
vtkSPtrNew( cells, vtkCellArray );
vtkIdType bottom_ptIds1[3] = { 2, 1, 0 };
cells->InsertNextCell( 3, bottom_ptIds1 );
vtkIdType bottom_ptIds2[3] = { 0, 3, 2 };
cells->InsertNextCell( 3, bottom_ptIds2 );
vtkIdType top_ptIds1[3] = { 5, 6, 4 };
cells->InsertNextCell( 3, top_ptIds1 );
vtkIdType top_ptIds2[3] = { 4, 6, 7 };
cells->InsertNextCell( 3, top_ptIds2 );
vtkIdType left_ptIds1[3] = { 0, 4, 3 };
cells->InsertNextCell( 3, left_ptIds1 );
vtkIdType left_ptIds2[3] = { 7, 3, 4 };
cells->InsertNextCell( 3, left_ptIds2 );
vtkIdType right_ptIds1[3] = { 5, 2, 6 };
cells->InsertNextCell( 3, right_ptIds1 );
vtkIdType right_ptIds2[3] = { 1, 2, 5 };
cells->InsertNextCell( 3, right_ptIds2 );
vtkIdType front_ptIds1[3] = { 0, 1, 4 };
cells->InsertNextCell( 3, front_ptIds1 );
vtkIdType front_ptIds2[3] = { 1, 5, 4 };
cells->InsertNextCell( 3, front_ptIds2 );
vtkIdType back_ptIds1[3] = { 2, 7, 6 };
cells->InsertNextCell( 3, back_ptIds1 );
vtkIdType back_ptIds2[3] = { 3, 7, 2 };
cells->InsertNextCell( 3, back_ptIds2 );
points->InsertNextPoint( -1, 4, 1 );
vtkIdType extraCell1[3] = { 8, 5, 4 };
cells->InsertNextCell( 3, extraCell1 );
points->InsertNextPoint( 3, 2, -1 );
points->InsertNextPoint( 3, 4, -1 );
vtkIdType extraCell2[3] = { 9, 10, 6 };
cells->InsertNextCell( 3, extraCell2 );
vtkSPtrNew( result, vtkPolyData );
result->SetPoints( points );
result->SetPolys( cells );
result->BuildCells();
result->BuildLinks();
result->Modified();
// ==================== Finish: cube ====================
vtkSPtrNew( mapper, vtkPolyDataMapper );
mapper->SetInputData( result );
vtkSmartPointer<vtkSTLWriter> writer = vtkSmartPointer<vtkSTLWriter>::New();
writer->SetFileName( "cube.stl" );
writer->SetInputData( result );
writer->Write();
vtkSPtrNew( actor, vtkActor );
actor->SetMapper( mapper );
vtkSPtrNew( renderer, vtkRenderer );
renderer->AddActor(actor);
renderer->SetBackground( 0, 0, 0 );
vtkSPtrNew( renderWindow, vtkRenderWindow );
renderWindow->AddRenderer( renderer );
vtkSPtrNew( renderWindowInteractor, vtkRenderWindowInteractor );
renderWindowInteractor->SetRenderWindow( renderWindow );
renderer->ResetCamera();
renderWindow->Render();
renderWindowInteractor->Start();
return 0;
}
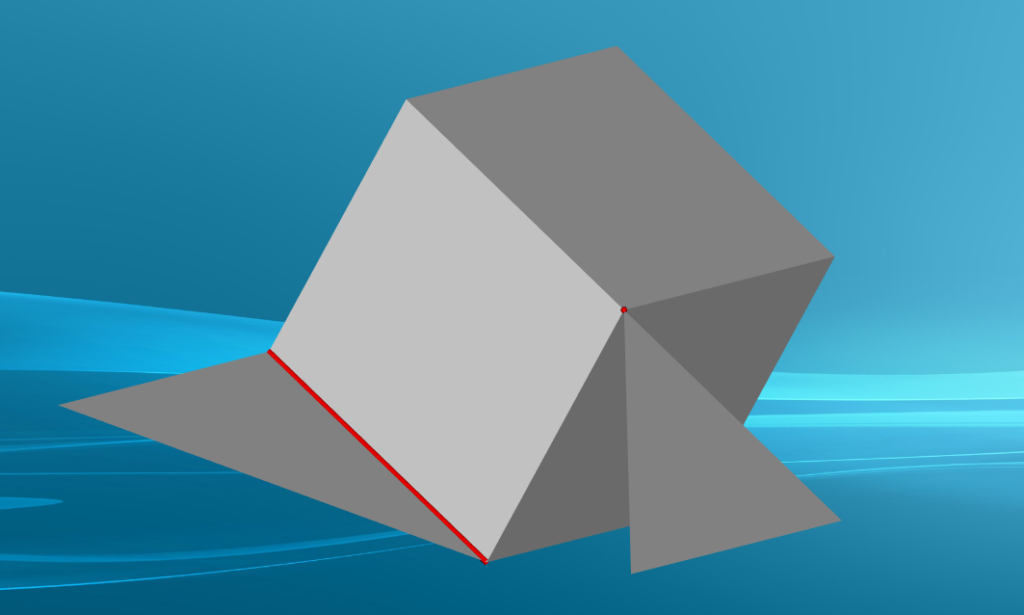
The model file had been uploaded to server:
https://www.weiy.city/Download/non-manifold-model.stl
You can handle these non-monifold elements in the new function non-manifold edge/point in this web tool.
Here is demo video:
Tool URL: